Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial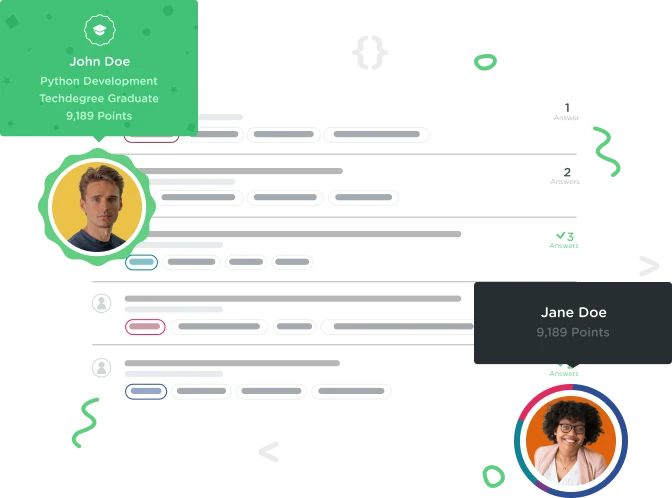
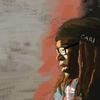
Carl Sergile
16,570 PointsNot getting the Alert Dialog to Show. No errors in code.
Hey so I did exactly as the video instructed. I couldn't get the Alert Dialog Box to show. My app does run and it shows everything else.
Here is my code - MainActivity.java :
package creator2earth.com.stormy;
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.util.Log;
import java.io.IOException;
import okhttp3.Call; import okhttp3.Callback; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response;
public class MainActivity extends AppCompatActivity { public static final String TAG = MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// API key from DarkSky.net
String apiKey = "1a1c1ff4b2f4e7099ad64aa29c61aa3c";
double latitude = 999.9; //37.8267;
double longitude = -122.4233;
String forecastUrl = "https://api.darksky.net/forecast/1a1c1ff4b2f4e7099ad64aa29c61aa3c/" +
apiKey +
"/" + latitude + "," + longitude;
/*
1. When enqueue method is called its saying callback when you are done.
2. When call is finished. The onResponse() callback method from the phone is executed.
3. If there is an error then the onFailure method would work.
*/
// Request a client with OKHTTP.
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() { // the equeue call back method.
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
// Try / catch block. Try to see if response is successful. If so log it.
try {
Log.v(TAG, response.body().string());
if(response.isSuccessful()) {
} else {
alertUserAboutError(); // Make sure to put this method in the main activity and not inside the anonymous class.
}
} catch (IOException e) { // If it fails with an IO exception, then run this code instead.
Log.e(TAG, "Exception caught", e);
} // Otherwise proceed as normal.
}
});
Log.d(TAG, "Main UI code is running!");
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
AlertDialogFragment.java
package creator2earth.com.stormy;
// make sure to import DialogFragment using android.app instead of android.support.
import android.app.Dialog; import android.app.DialogFragment; import android.content.Context; import android.os.Bundle; import android.support.v7.app.AlertDialog;
public class AlertDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity(); // normally 'this' is use but getActivity() gets the activity that the Dialog Fragment is created in.
AlertDialog.Builder builder = new AlertDialog.Builder(context)
.setTitle(context.getString(R.string.error_title)) // This sets the Dialogs title.
.setMessage(context.getString(R.string.error_message))
.setPositiveButton("OK", null); //This sets the okay button
// This creates the builder and sets it in a new variable called dialog.
return builder.create(); // Returns dialog
}
}
Then lastly my strings.xml:
<resources> <string name="app_name">Stormy</string> <string name="action_settings">Settings</string> <string name="error_title">Oops! Sorry!</string> <string name="error_message">There was an error. Please try again.</string> <string name="error_ok_button_text">OK</string> </resources>
Any help on this would be great as I am not getting any error messages so I don't really know whats going on. Thanks.
2 Answers

Seth Kroger
56,413 PointsYou forgot to wrap the call with runOnUiThread(), or didn't get to that part of the videos yet.

Michael Nock
Android Development Techdegree Graduate 16,018 PointsI was having the same issue. It appeared to load the page when the URL was correct, but didn't enter the onResponse method when the URL was incorrect. For me, it turned out to actually be running the onFailure method, which i believe is your case too. You've got your API key listed twice in the URL. Once hardcoded and once as a variable. Remove the first hardcoded one and you should be good to go.
So try placing your alertUserAboutError method in the onFailure method and you should see it. Then fix your URL and it should work in the else block of the onResponse method :)
You also might not wanna post your API key publicly, others could copy it and use it themselves. For your free key it's probably not an issue (unless you actually planned on using the app yourself and run out of free requests). But in the future...