Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial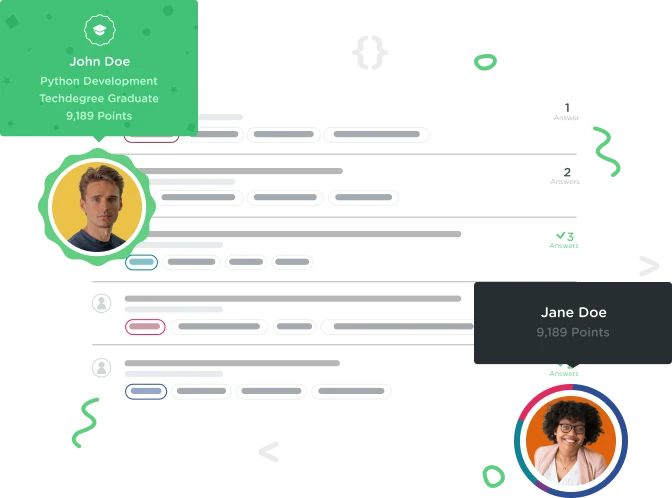

Jake Nagle
2,942 PointsNot getting the right output and I'm not sure why
Sorry, I already know this is going to be a long question. I'm working on the java course and am currently working on the hangman game. So my code is compiling but when I run it, it should return this:
Try to solve: ----------
but instead does this:
Try to solve: treehouse
So clearly the functions that change the letters to dashes aren't working. I can't figure out why and I have watched the video multiple times. Any help or advice is great, thanks! Here's the code for the game class:
public class Game {
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0); {
display = letter;
}
progress += display;
}
return progress;
}
}
Here's the code for the Hangman class:
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
prompter.displayProgress();
boolean isHit = prompter.promptForGuess();
if (isHit) {
System.out.println("We got a hit!");
} else {
System.out.println("Whoops that was a miss");
}
prompter.displayProgress();
}
}
And finally for the Prompter class:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
public void displayProgress() {
System.out.printf("try to solve: %s\n", mGame.getCurrentProgress());
}
}
2 Answers
Kathryn Ann
10,071 PointsHi Jake - In the getCurrentProgress() method in the Game class, there's an extra semicolon after the parentheses in the if statement:
public String getCurrentProgress() {
String progress = "";
for (char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0); {
display = letter;
}
progress += display;
}
return progress;
}

Jake Nagle
2,942 PointsThanks Kathryn! I'm surprised that i missed that and that I didn't get an error.