Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial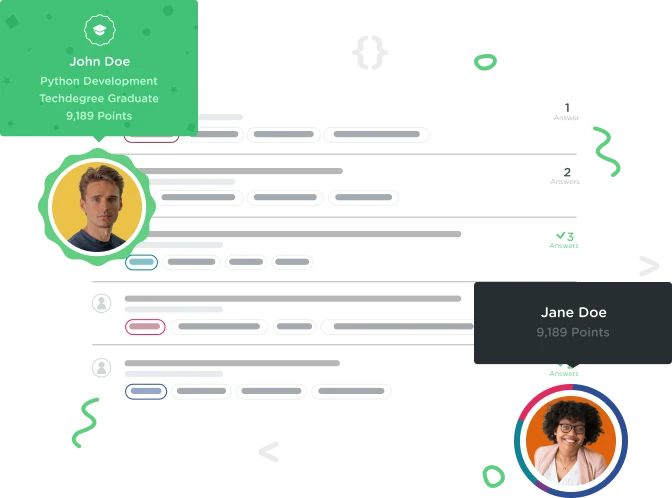
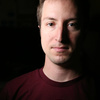
Yohanan Braun-Feder
6,101 PointsNot knowing how to solve a problem. What to do?
I have more or less plowed through beginner HTML and CSS. JavaScript was where things got interesting, or should I say frustrating. I find it hard to figure out how to translate the tasks at hand to actual code - what to use in order to accomplish the job and how to plan it out. It's really getting to me and I'm having trouble getting past this point. What would be a good course of action?
3 Answers
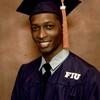
Dane Parchment
Treehouse Moderator 11,077 PointsStop and Think: I know that this sounds quite obvious but really, just stop for a moment and think about what is being asked of you. Sometimes people will either overthink a simple problem, or not meet the requirements of a difficult problem. I recommend reading what is specifically being asked of you to do. Do you need to display content to a web page, display info to the console, loop through a bunch of html elements? These are the things that you should be figuring out that you need to do.
Once you figure out what you need to be doing, now you need to figure out if this is something that you can figure out with your current skillset. If you are being asked to create the back-end server code for Nodejs to connect to MongoDB, and all you know how to do is add the script element to an html document then you know that maybe you need to review things. However, if they are asking you to simply print a message to the screen and you know how to add a script to the html document and about the document.write command then you know that you should be fine. This is a pivotal step because it will help you determine if you need to review concepts you may not know or if you can dive in and solve the problem. Break it up into smaller parts. Once you know that you can solve the problem break it into smaller, more manageable parts. This is a great opportunity to practice using code comments and your psuedocode and algorithm skills. All a program is a list of commands that you are giving the computer, so sometimes all you need to do is tell the computer how you would solve the problem. If the problem is complex just break it into smaller parts, similiar to how you would solve a math word problem in school. Here is how I would do it.
- Look for anything that you can abstract into a variable.
- If math is involved find out if the language that you are programming in has methods to make this faster (ie. cos, sin, tan functions)
- Determine if loops, or conditional statements are necessary. Determine if data structures need to be used (a data structure is a way for a programming language to store and move around data ie. an array in javascript)
- Figure out if the language has pre-made methods that will make life easier, or if you have to create your own.
- Once these are all figured out, dive in and solve the problem!
If you still can't figure it out or are having problems the programming community is a friendly bunch and though they can be cynical or very blunt at times, they will almost always go out of their way to help you solve your problem. So check out forums like this one or Q&A sites like StackOverflow to find help for your problems. But if you want to learn try not to ask for the actual answer to your problem and instead try to see if they can give you a step in the right direction so that you can solve the problem yourself. I mean you can't learn if everyone else if giving you the answer.
Well now that I have shown you the steps. let me actually walk you through myself solving a popular programming question: Fizzbuzz (generally asked on a lot of programming/software engineering interviews):
Here is the question (we will solve it with JavaScript but you can use any language you desire):
"Write a program that prints the numbers from 1 to 100. But for multiples of three print “Fizz” instead of the number and for the multiples of five print “Buzz”. For numbers which are multiples of both three and five print “FizzBuzz”."
Ok so let's begin shall we? So let's start with step 1. I am going to stop and think about what is being asked of me. I have to write a program that prints the numbers 1 - 100, and if the number is divisible by 3 it should display fizz and not the number, or if it is divisible by 5 it should be buzz that is displayed and finally if both are divisible then print fizzbuzz.
Great now onto step 2: I know that I need to have a basic grasp of math and what divisibility is (simply a number can be divided by another number and return a whole number (1, 2, 3...etc.) instead of a fraction or improper number (1.234, 1.442, etc.), javascript has a math operator called modulos (%) that i can use to make finding divisibility easier if the number1 % number2 == 0 then it is divisible otherwise it isn't. I also know that in order to print the numbers between 1 and 100 I need to use a loop, which I also know how to create. Finally I see that I have to make checks of whether or not the number is divisible by others, so conditional statements shall also be used.
Finally it is time to get coding but before I do that, let me write an outline of code (my psuedocode and algorithm) to solve this process using javascript code comments:
//create a loop that loop that starts at 1 and increased until it reaches 100
//if the value of the current number of the loop is divisible by both numbers display fizzbuzz
//else if it is divisible by 3 only display fizz
//else if it is divisible by 5 only display buzz
//otherwise just print the number
//create a loop that loop that starts at 1 and increased until it reaches 100
for(var i = 1, j = 0; i <= 100; i++) {
//if the value of the current number of the loop is divisible by both numbers display fizzbuzz
if(i % 3 == 0 && i % 5 == 0) {
document.write("FizzBuzz" + "<br>");
}
//else if it is divisible by 3 only display fizz
else if(i % 3 == 0) {
document.write("Fizz" + "<br>");
}
//else if it is divisible by 5 only display buzz
else if(i % 5 == 0) {
document.write("Buzz" + "<br>");
}
//otherwise just print the number
else {
document.write(i + "<br>");
}
Hopefully this workflow will help you understand how to go about solving problems if you still need help. Let me know!
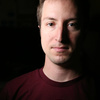
Yohanan Braun-Feder
6,101 PointsThanks for the look behind the scenes! I appreciate the depths you have taken to answer my question. I will implement it with my current project.
YB
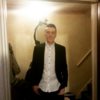
James Barrett
13,253 PointsI have a similar problem. I always feel like I don't know what to do for the code challenges. However when the code was given to me I can read it perfectly. I guess practice makes perfect and to not over think it.