Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial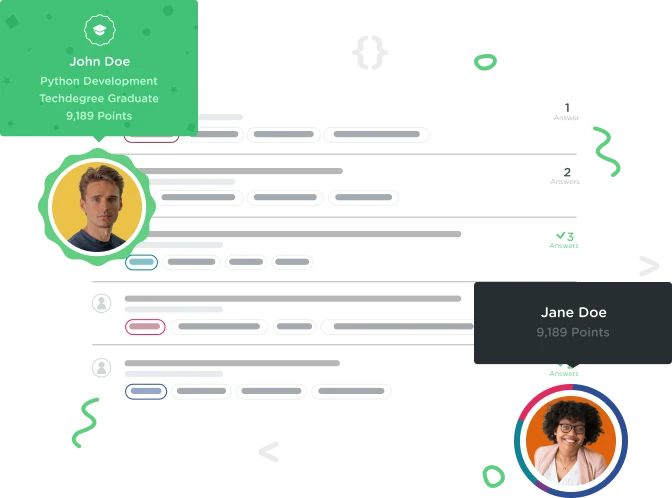

samarth sharma
1,758 PointsNot operator
Declare a constant named isWinner and assign the results of a comparison operation to check whether the player has won or not. If the total score is not 10, then the player has won, otherwise he or she has lost. (Hint: Use the NOT operator)
3 Answers

Chris McKnight
11,045 PointsYou can use the not operator, !
with NSNumber#isEqualToNumber
. Alternatively, you could technically use the does not equal operator. Just be sure you compare primitives to primitives or use a method that will compare objects such as NSNumber#isEqualToNumber
.
If you use isEqualToNumber
, you will have to wrap any primitive numbers with NSNumber
See example code below with comments
var scoreLimit = 10
var a = 5
var b = 2
var c = NSNumber(int: 10)
if !c.isEqualToNumber(NSNumber(integer: scoreLimit)) {
print("C does not equal \(scoreLimit)")
}
if a != scoreLimit {
print("A does not equal \(scoreLimit)")
}
if b != scoreLimit {
print("B does not equal \(scoreLimit)")
}

Martin Wildfeuer
Courses Plus Student 11,071 PointsJust a small addition: if you wanted to use the ! operator as a prefix, you could also write
if !(a == scoreLimit) {
print("A does not equal \(scoreLimit)")
}
@samarth sharma: The key to this challenge is indeed the !=
(not equal) operator, though :)

Chris McKnight
11,045 PointsMartin Wildfeuer Many ways to solve the problem :)
The hint leads towards !(a == scoreLimit)
or !a.isEqualToNumber(NSNumber(integer: scoreLimit))
IRL, it doesn't matter as long as it's readable and correct.

samarth sharma
1,758 Pointshey guys thanks for your prompt reply.. i was stuck initially with the following code:
let isWinner = totalScore
totalScore == 10
i was getting an error. i re-read the question multiple times and tried to figure out the logic.
finally, i wrote the following code and was correct
let isWinner = totalScore != 10
thanks for the answers again as they showed me the alternative ways to execute the same command :)

Martin Wildfeuer
Courses Plus Student 11,071 PointsGood job, well done! :)
Martin Wildfeuer
Courses Plus Student 11,071 PointsMartin Wildfeuer
Courses Plus Student 11,071 PointsHey there! Please post what you have tried or try to explain what you are struggling with. The community here is keen to help, but the idea is to ask questions. Posting the solutions to an assignment should be the very last step of an explanation :)