Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial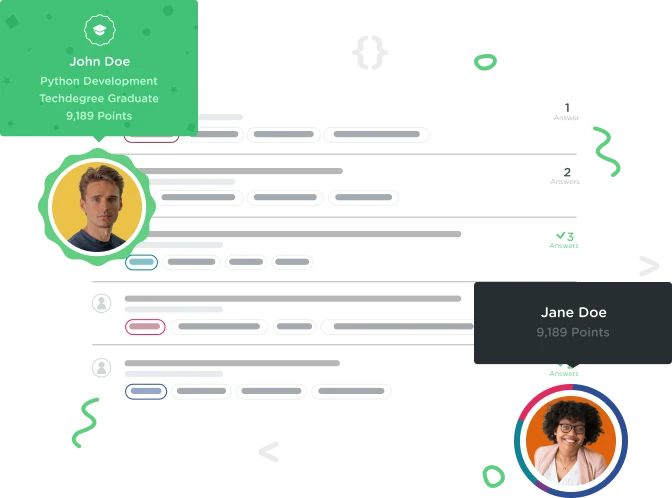
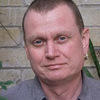
Unsubscribed User
4,479 Points'Not' operator confusion
I am confused by the 'not' operator - !
I know that it reverses the condition of a variable from 'true' to 'false'. But the way Dave explains it in the 'For Loops' videos makes it sound like the '!' operator reverses the CURRENT condition of the variable saved elsewhere in the code.
So, using his example,
var correctGuess = false;
Would
( ! correctGuess )
reverse the CURRENT condition of the variable 'correctGuess' to 'true', or will it always set the variable condition to 'NOT true', i.e. false?
3 Answers
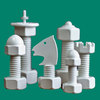
Steven Parker
229,744 PointsThe not operator ("!
") returns the opposite of the current value.
So, for example, if correctGuess
is true
, then !correctGuess
would be false
.
But if correctGuess
is false
, then !correctGuess
would be true
.
Either way, correctGuess
itself is not changed by using the operator.
Here's a code example:
correctGuess = true;
if (correctGuess)
alert("correctGuess is true"); // you will see this message
else
alert("correctGuess is false");
if (!correctGuess)
alert("correctGuess is NOT true");
else
alert("correctGuess is NOT false"); // you will see this message
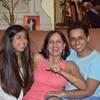
Sameer Dewan
Courses Plus Student 13,145 PointsLet's use an example..recall first that // + text on a line indicate comments that do not affect the code
var switch = true;
//documentation
//while switch is true, switch is on.
//while switch is false, switch is off.
therefore, if console.log(switch) in the developer console, I will be returned with 'true'. But! If I console.log(!switch), I will be returned with 'false'.
These conditionals can be used to make logical basic decisions in js.
ex:
function switchToggle() {
if(switch) {
console.log('my light is on!')
}
if(!switch) {
console.log('my light is off!!')
}
In reality, I would've used an else statement here, but what the above is saying is: If the variable 'switch' is 'true', log this to the console. If 'switch' is assigned to false, log this alternative to the console.
You would be able to control your "switch" from the console by first re-assigning 'switch' to equal false, and then running the function I've provided.
switch = false;
switchToggle();
Hope that helps.
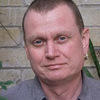
Unsubscribed User
4,479 PointsThanks for your replies.
Steven, that's very clear and answers my question. Thanks.