Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial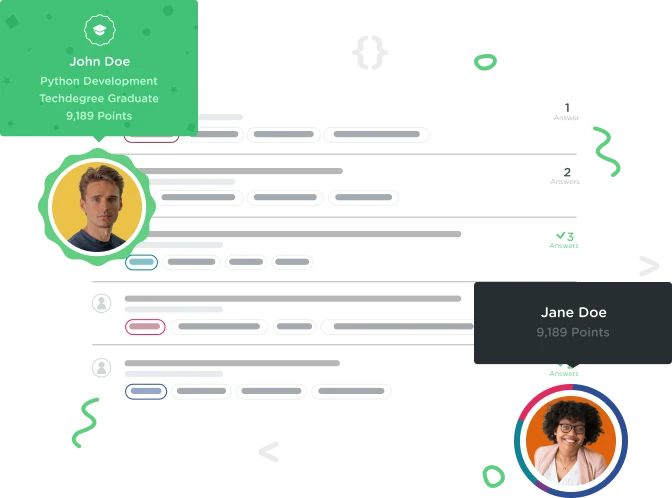
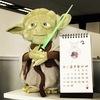
michaellesniak
10,443 PointsNot passing the code challenge: iOS AutoLayout
Can anyone see what's missing? I keep getting a "Bummer! Try again!" message. Thanks in advance.
class MyViewController: UIViewController {
let sampleView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(sampleView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
sampleView.translatesAutoresizingMaskIntoConstraints = false
setupSampleViewConstraints()
// Add constraints below
}
func setupSampleViewConstraints() {
let CenterX = NSLayoutConstraint(item: sampleView, attribute: .centerX, relatedBy: .equal,
toItem: view, attribute: .notAnAttribute, multiplier: 1.0, constant: 0.0)
let CenterY = NSLayoutConstraint(item: sampleView, attribute: .centerY, relatedBy: .equal,
toItem: view, attribute: .notAnAttribute, multiplier: 1.0, constant: 0.0)
let Height = NSLayoutConstraint(item: sampleView, attribute: .height, relatedBy: .equal,
toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 50.0)
let Width = NSLayoutConstraint(item: sampleView, attribute: .width, relatedBy: .equal,
toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 50.0)
view.addConstraints([CenterX, CenterY, Height, Width])
}
}
1 Answer
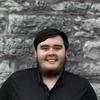
Michael Hulet
47,912 PointsYour problem here is that you're specifying .notAnAttribute
in the 2nd attribute
parameter in your constraints to center along both the X and y axes. For these, the centering attribute is an attribute, and you'll need to specify the proper attribute twice to keep the center of sampleView
equal to the center of its superview. The code would look something like this:
let centerX = NSLayoutConstraint(item: sampleView, attribute: .centerX, relatedBy: .equal,
toItem: view, attribute: .centerX, multiplier: 1.0, constant: 0.0)
let centerY = NSLayoutConstraint(item: sampleView, attribute: .centerY, relatedBy: .equal,
toItem: view, attribute: .centerY, multiplier: 1.0, constant: 0.0)
Between you and me, though, I like dealing with AutoLayout in code about as much as I like taking a swift kick to the face. I've never used it like this personally. I've always dealt with it via Interface Builder, and once with PureLayout, and both ways are much nicer
michaellesniak
10,443 Pointsmichaellesniak
10,443 PointsThanks so much! It's my first time so no reference, but it seems really tedious!!!