Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial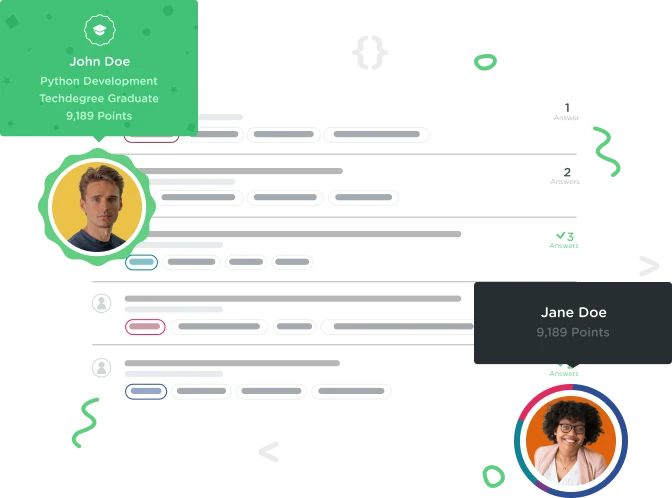

Ryan Weckesser
1,978 PointsNot printing both cards after incorrect guess.
Not sure why I am not getting both cards output (only one) when guessing incorrect match.
from cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ['Add', 'Boo', 'Cat', 'Dev', 'Egg', 'Far', 'Gum', 'Hut']
self.columns = ['A', 'B', 'C', 'D']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
location = f'{column}{num}'
self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word,random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
def create_grid(self):
# | A | B | C | D |
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, self.size + 1):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row) + ' |'
print(print_row)
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False
def check_win(self):
for card in self.cards:
if card.matched == False:
return False
return True
def check_location(self, time):
while True:
guess = input(f"What's the location of your {time} card? ")
if guess.upper() in self.locations:
return guess.upper()
else:
print("That's not a valid location. It should look like this: A1")
def start_game(self):
game_running = True
print('Memory Game')
self.set_cards()
while game_running:
self.create_grid()
guess1 = self.check_location('first')
guess2 = self.check_location('second')
if self.check_match(guess1, guess2):
if self.check_win():
print('Congrats!! You have guessed them all!')
self.create_grid()
game_running = False
else:
input('Those cards are not a match. Press enter to continue')
print('GAME OVER')
if __name__ == '__main__':
game = Game()
game.start_game()
1 Answer
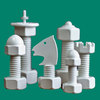
Steven Parker
230,274 PointsThe indentation of the "return" on line 61 places it inside the loop, causing the loop to end immediately after printing the first card.
For future questions, a better way to share the code of a large and/or multi-file project is by making a snapshot of your workspace and posting the link to it here.
Ryan Weckesser
1,978 PointsRyan Weckesser
1,978 PointsThank you!
Sorry for the wall of code.