Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial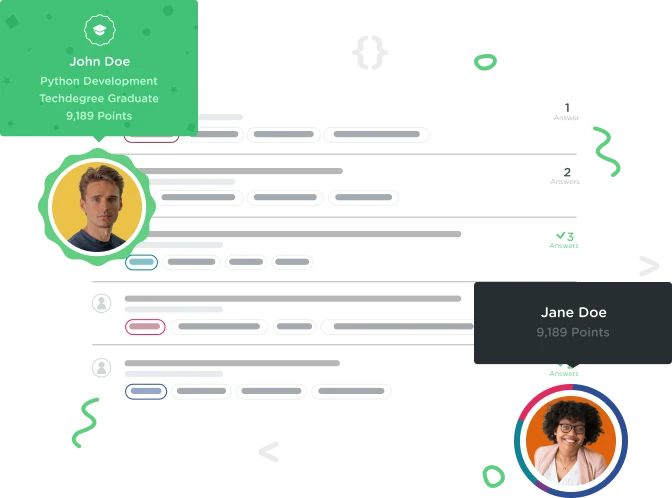
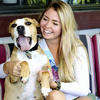
Kennedi Armstrong
883 PointsNot printing "You win!..."
When I guess the word right, the "You win!..." message does not print.
import random
make a list of words
words = [ 'apple', 'banana', 'orange', 'coconut', 'strawberry', 'lime', 'grapefruit', 'lemon', 'kumquat', 'blueberry', 'melon' ]
while True: start = input("Press enter/return to start, or enter Q to quit") if start.lower() == 'q': break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
# draw spaces
# draw guessed letters, spaces, and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print("Strikes: {}/7".format(len(bad_guesses)))
print('')
#take a guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only print letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}.".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess it! My secret word was {}.".format(secret_word))
2 Answers

Jimmy Smutek
Python Web Development Techdegree Student 6,629 PointsI had the same issue, Kennedi.
The problem is that strings with multiple occurrences of a single letter will never make it past these lines:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
For example, if the string being evaluated is apple
, and on the first guess the user enters p
, then len(good_guesses)
will return 1, because we're only adding 1 occurrence of p
to the list. It makes sense on the surface, because there's only been 1 good guess thus far - but we're set up to fail because we're evaluating the amount of good guesses against the amount of letters in the target.
So, in the case of apple
, when the user reaches the end, good_guesses
will contain ['a' , 'p', 'l', 'e']
, len(good_guesses)
will return 4, while len(list(secret_word))
returns 5.
I'm sure there's a more elegant solution, but I was able to get around this by using count()
and then adding each occurrence of the letter to the list before counting.
# check for multiple occurrences of guess
occurrences = secret_word.count(guess)
# += will add each occurrence as a separate instance -
good_guesses += (guess * occurrences)
# now check the length
I couldn't get it to work with append()
, because it added the occurrences on as an additional list, but +=
seemed to do the trick. Here's my working code - hope this is helpful!
import random
# make a list of words
words = [
'apple',
'banana',
'coconut',
'strawberry'
]
while True:
start = input("Press enter to start, or Q to quit: ")
if start.lower() == 'q':
break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
# draw spaces guessed letters and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
# take guess
guess = input('Guess a letter: ').lower()
if len(guess) != 1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
# check for multiple occurrences of guess
occurrences = secret_word.count(guess)
print('Guess occurs {} times in the secret word'.format(occurrences))
# list.append() adds as a list, ie. ['a', ['pp'], 'l', 'e']
# += will add each occurrence as a separate instance -
good_guesses += (guess * occurrences)
# now check the length
if len(good_guesses) == len(list(secret_word)):
print("you win! The word was {}".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You did not guess it! My secret word was {}".format(secret_word))

Mike Wagner
23,559 PointsYou had a bit of a formatting issue in the way you posted your code in the question, so I'm not sure what your problem actually is. Once I took it into my private environment and adjusted the tabbing to what I thought would work I was left with a functional program that worked as expected. I'll add it here so you can have a look through it and try to find the difference that makes your version fail, but I'm guessing it has something to do with a misplaced or missing indent.
import random
words = [ 'apple', 'banana', 'orange', 'coconut', 'strawberry', 'lime', 'grapefruit', 'lemon', 'kumquat', 'blueberry', 'melon' ]
while True:
start = input("Press enter/return to start, or enter Q to quit")
if start.lower() == 'q': break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
# draw spaces
# draw guessed letters, spaces, and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print("Strikes: {}/7".format(len(bad_guesses)))
print('')
#take a guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only print letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}.".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess it! My secret word was {}.".format(secret_word))
Sam Bui
878 PointsSam Bui
878 PointsThank Jimmy, I have the same problem and I baffle to try to understand what wrong with my code. Your explaination just spot on :)