Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial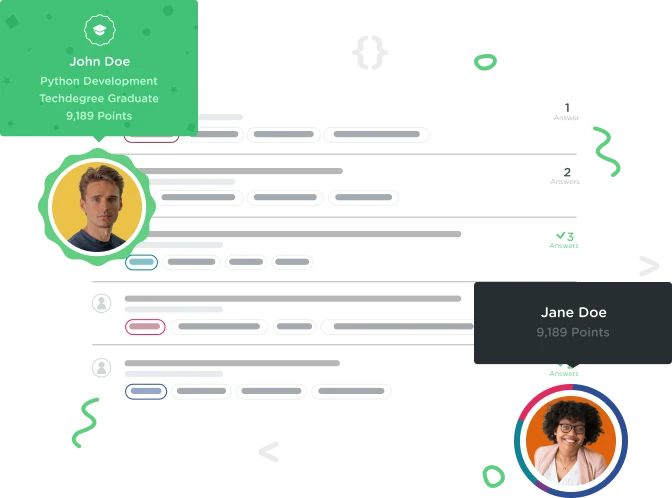
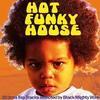
Jeff Burk
5,215 PointsNot quite sure what is going on...
Can someone please explain what this method is doing, especially the second part (VocabularyWord that = obj as VocabularyWord; return this.Word == that.Word;)
I am not understanding casting and the "as"
public override bool Equals(object obj)
{
if(!(obj is VocabularyWord))
{
return false;
}
VocabularyWord that = obj as VocabularyWord;
return this.Word == that.Word;
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object obj)
{
if(!(obj is VocabularyWord))
{
return false;
}
VocabularyWord that = obj as VocabularyWord;
return this.Word == that.Word;
}
}
}
3 Answers

andren
28,558 PointsAll objects in C# inherit from the object
class, because of that all object can be cast (treated as) an instance of the object
class. Because of that the Equals
method in your code can actually be passed any object regardless of what class it is an instance of.
The first thing the method does is to check if the object passed in is not an instance of VocabularyWord. If it isn't then it obviously won't be equal to the VocabularyWord object that the equal
method is inside of.
if(!(obj is VocabularyWord)) // Check if passed in obj is not an instance of VocabularyWord
{
return false; // If that is the case return false right away.
}
If the passed in object is an instance of VocabularyWord
that has been cast as an object
then you need to cast it back to being a VocabularyWord
. This is needed because while it is cast as an object
you can only access methods and properties that would be present on an actual object
class. Any method or property that exist only on VocabularyWord
objects won't be accessible without the object being cast to the right type.
VocabularyWord that = obj as VocabularyWord;
The as
keyword is just a simple way of telling C# to cast obj as a VocabularyWord
instance. Then you assign that instance to a variable called that
.
After all that work it's time for the actual equality comparison, which is the main point of the Equals
method. this.Word
refers to the Word
property found within the object that the Equals
method belongs to, while that.Word
refers to the Word
property that belongs to the object that was passed in to the function.
After comparing the two Word
properties the function returns the result.
return this.Word == that.Word; // Compare this.Word == that.Word then return result (true or false)
One thing that is important to note about casting objects, which tends to confuse people, is that casting an object does not actually change the object itself. It essentially only affects how C# treats the object. So when a VocabularyWord
instance gets cast as an object
instance it does not actually change anything about the VocabularyWord
instance, it only makes it so that C# will treat it exactly as if it were an instance of object
. Which is why you can cast an object back and forth without losing any data.

Tobias Karlsson
2,751 PointsOne thing to mention about casting with as
is that it will return null
if the cast is not successful and not an exception. That in turn means that you can safely do the comparison
this.Word == that.Word
even if that.Word
is null
, that will return false
.
Here you can read about the two operator keywords:
The is operator
The as operator
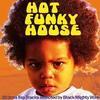
Jeff Burk
5,215 Pointsandren and Tobias, thank you both for the expanded explanation! This helped.