Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial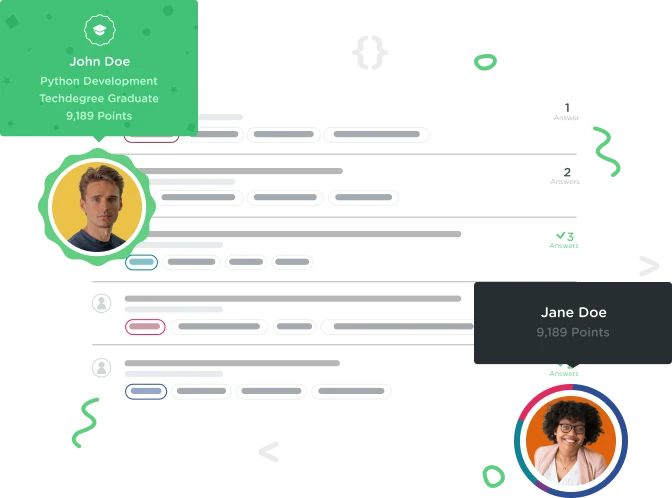

Craig Watson
27,930 PointsNot quite understanding the difference between "return" / "echo" when used with functions
Hi, this part of the challenge has me a bit lost ??
At which point if any do i need to the reference the array containing the number to be added up ??
1 Answer

Aaron Graham
18,033 PointsYou would reference your array inside the function the challenge asks you to build:
function mimic_array_sum($array) {
//you need something to track the running sum of your array
$total = null;
//reference the array containing number here.
//use a foreach loop to iterate through the array
//inside the loop, add the numbers to your running total
foreach($array as $number) {
$total += $number;
};
//return the total from the function
return $total
}
Once you have your function, you can use it by calling it with an array as the argument, and store the returned value in another variable:
$sum = mimic_array_sum($palindromic_primes);
and then echo the returned value that is now stored in $sum
:
echo $sum;
Does that make sense?
Craig Watson
27,930 PointsCraig Watson
27,930 PointsHi Aaron,
Im getting a better handle on the way it needs to be set out and how it loops through to count items in the array but how do I manage to get the total of the number in the array added together :s
I know you mentioned tracking the running total using $total = null; I wasnt sure how this would calculate the/ have access to the array ?
Thanks for your help ..............(again!!)
Aaron Graham
18,033 PointsAaron Graham
18,033 PointsCraig Watson - No problem.
The way the $total variable and the foreach loop works is as follows:
Consider the function in question:
When you call this function, it is going to loop through each element of the array, and on each iteration, it will add the current $number to $total. Say for example, you called it like this:
There are three values in the array, so the foreach loop will run three times. On the first iteration, $total is still set to null, so you basically have $total = null + 1. On the next iteration, $total contains the result of the last iteration of the loop, in this case 1, so you have $total = 1 + 2. At this point, $total is equal to 3. When the last iteration runs, you again add the current value of $total to the current $number and reassign that value to $total, which works out to be $total = 3 + 3. Once the foreach loop has finished iterating through all the elements of the array, program execution continues to the next line, which simply says
return $total
. The $total that is being returned here is the variable that contains the accumulated sums from the foreach loop. This is the return value of the function, and can be assigned to other variables. In this example this is the$sum = mimic_array_sum($my_array);
expression. It should be pointed out that, when the function returns, it is destroyed and all of its variables with it. The consequence of this is that you can call the function as many times as you want and always get predictable results. For example:will always give you the same result. I point this out to illustrate that the $total that is declared inside the function is thrown away after the function executes, and a new variable is created when you call the function again. That is sometimes a source of confusion as well.
Hope that helps.
Craig Watson
27,930 PointsCraig Watson
27,930 PointsHurray !!! I've Got It,
Really big thanks for helping me on that one I'm glad I not only passed the challenge but actually now hold a good understanding of the way it works and is set out!
Thanks a lot Aaron!!
Aaron Graham
18,033 PointsAaron Graham
18,033 PointsCraig Watson - Glad you got it! Happy coding!