Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial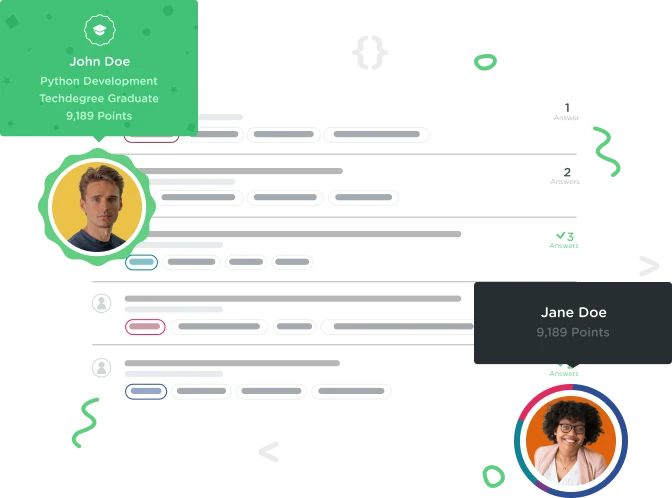

Timothy Van Cauwenberge
8,958 PointsNot really sure how to complete this challenge. Please help!
the challenge states: Let's write some functions to explore set math a bit more. We're going to be using this COURSES dict in all of the examples. Don't change it, though! So, first, write a function named covers that accepts a single parameter, a set of topics. Have the function return a list of courses from COURSES where the supplied set and the course's value (also a set) overlap. For example, covers({"Python"}) would return ["Python Basics"].
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(set1):
course_list = []
for course in COURSES:
10 Answers
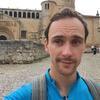
Christopher Kellett
7,053 PointsThis is the first time I've made a post to try to help somebody but I've just passed this challenge after quite a while so I thought I'd share my solution.
def covers(arg):
hold = []
for key, value in COURSES.items():
if value & arg:
hold.append(key)
return hold
covers({'Ruby'})
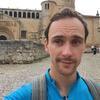
Christopher Kellett
7,053 Points...I can try but please be aware I'm still a newbie.
It really helped me to look at the python docs he recommends in 'Set Math'. I played around a bit in the shell to get the hang of the different methods and also watched that video a few times.
def covers(arg):
hold = []
for key, value in COURSES.items():
#key is the course names (e.g. Python, Ruby) and value is the sets{'Python', 'functions' etc.} that we want to compare
if value & arg:
#so value(set1).intersection(set2) - finds common items between sets
hold.append(key)
return hold
covers({'Ruby'})
As for the second challenge, you'll need to check out the method .issubset().
I can post the answer if you're struggling but I'm sure you'll get it once you get the hang of the common set operators (|, &, ^ and -) and have practised with the subset method in the shell.
Don't give up - it took me hours to get there! Hope this is helpful!

Abubakar Gataev
2,226 PointsI don't get why you are doing this: "if value & arg:" Where does he say in the task that you have to find all the common items between the 2 sets? please answer.
task: Let's write some functions to explore set math a bit more. We're going to be using this COURSES dict in all of the examples. Don't change it, though!
So, first, write a function named covers that accepts a single parameter, a set of topics. Have the function return a list of courses from COURSES where the supplied set and the course's value (also a set) overlap.
For example, covers({"Python"}) would return ["Python Basics"].

Omid Ashtari
4,813 Pointsdisregard, finally figured it out...not sure I fully understand, but this passed the test:
def covers_all(single_set):
new_list = []
for key, value in COURSES.items():
if set(single_set).issubset(value) :
new_list.append(key)
return new_list
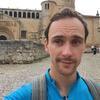
Christopher Kellett
7,053 PointsNice one , that's the same way I did it!

Aquiba Benarroch
2,875 PointsExactly the same methodology but using issuperset instead.
def covers_all(topics):
all_courses = []
for keys, values in COURSES.items():
if values.issuperset(topics):
all_courses.append(keys)
return all_courses
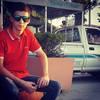
Robert Stepanyan
2,297 PointsAn easier way to understand (We are outputting answer like a list item because the answer should be like " ['Python Basics'] "
def covers(argument):
answer = []
for key, value in COURSES.items():
if value.intersection(argument):
answer.append(key)
return answer

Sagar Jain
2,140 PointsThanks for it

Dean Vollebregt
24,583 PointsHere is a solution using set operators instead of lists.
def covers(arg):
solution = []
for key, value in COURSES.items():
if set(arg) & set(value):
solution.append(key)
return(solution)

Dean Vollebregt
24,583 Pointscan treehouse fix the buggy copy and paste thing going on

Omid Ashtari
4,813 Pointshey @chriskellett, I sure could use the help on task 2...am stuuuuccckkk - thx

Samuel Havard
6,650 PointsThis is a bit late and I'm sure you figured it out by now, but I'll post for anyone else who comes looking for help.
For part 2, look to see if you arguments are a subset of the key words in COURSES:
def covers_all(input_set):
course_list = []
for course, key_word in COURSES.items():
if input_set.issubset(key_word):
course_list.append(course)
return course_list

bowei zhou
1,762 Pointsdef covers(a): b = [] for k,v in COURSES.items(): if v & a: b.append(k) return b

Ibrahim Khan
3,353 Pointsdef covers(argument):
course_list = []
for key, value in COURSES.items():
if argument.intersection(value):
course_list.append(key)
return course_list
I used this code and it seems to work.
I did a bunch of tests in workspaces. The problem is the code works only if the argument is given in the same format as the string it has to search for in the courses dictionary's set. For eg. inputing {"strings"} will return ['Python Basics', 'Java Basics', PHP Basics', 'Ruby Basics'], or inputting {"Python"} will return ['Python Basics'].
Lets say we input {"Strings"} instead of {"strings"} or {"python'} instead of {"Python"}, the code returns an empty list: []. Its because the format of the string in argument doesn't match the format of the course in the dictionary while it runs the loop, and thus doesn't append to the empty list we created.
What is the work around for this so the string format doesn't matter?

John Perry
Full Stack JavaScript Techdegree Graduate 41,012 Pointsdef covers(my_topics):
courses = []
for course, course_topics in COURSES.items():
if course_topics.intersection(my_topics): #equivalent to course_topics & my_topics
courses.append(course)
return courses
Here's the answer with better naming conventions so that it's easier to understand what is going on.
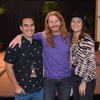
Ty Yamaguchi
25,397 PointsHere's how I did it, folks!
def covers(topic_set):
course_list = []
for course, topics in COURSES.items():
if topics & topic_set:
course_list.append(course)
return course_list
def covers_all(topic_set):
course_list = []
for course, topics in COURSES.items():
if not topic_set - topics:
course_list.append(course)
return course_list
Timothy Van Cauwenberge
8,958 PointsTimothy Van Cauwenberge
8,958 PointsThanks for the help. However, I'm having trouble understanding sets, do you think you could breakdown your code for me? I'm also having trouble with the next part of the challenge.
OK, let's create something a bit more refined. Create a new function named covers_all that takes a single set as an argument. Return the names of all of the courses, in a list, where all of the topics in the supplied set are covered.
For example, covers_all({"conditions", "input"}) would return ["Python Basics", "Ruby Basics"]. Java Basics and PHP Basics would be exclude because they don't include both of those topics.
Kimberly Vallejo
31,670 PointsKimberly Vallejo
31,670 PointsThank you so much for this. I struggled with this one for a long time.
Amber Readmond
7,861 PointsAmber Readmond
7,861 PointsThank you thank you thank you thank you Christopher Kellett!!!!!! I've been stuck on this challenge for literal days! I can actually understand your code- you rock! :)