Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial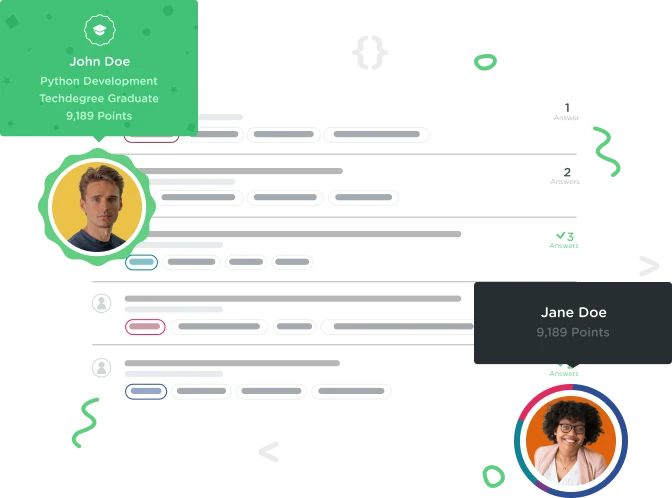

Kris oi
4,910 PointsNot really understanding
Dave had set the correctGuess = false
do(prompt) if (prompt was the same as randomNumber) change correctGuess to true... otherwise loop while(!correctGuess)
since correctGuess as initially false wouldn't !correctGuess in the while condition be true ... then shouldn't the loop continue as long as the answer true and stop if it was false
5 Answers
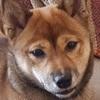
Katie Wood
19,141 PointsHi there,
Given something like this (your code from above):
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor (Math.random () * upper) + 1;
return num;
}
do {
guess = prompt('I am thinking of a number between 1 and 10. What is it?');
guessCount+=1;
if (parseInt(guess) === randomNumber) {
correctGuess = true;
}
} while(!correctGuess)
document.write('<h1> You guessed the number!</h1>');
document.write ('It took ' + guessCount + ' tries to guess the right number');
I think it might be best to explain with a focus on the correctGuess variable. As you've pointed out, it is set to false at the beginning. Think of this variable as an indication of whether the user has guessed the correct number or not. Since they haven't guessed anything yet, they have not - so, false.
We've generated the number at this point, so we're ready to guess. Enter the do-while loop:
-
The user is asked to guess, and the guess count increases.
guess = prompt('I am thinking of a number between 1 and 10. What is it?'); guessCount+=1;
-
The guess is compared to the number (randomNumber). If the user guesses correctly, correctGuess would be set to true, indicating that they have guessed the correct number. Otherwise, it stays false because they have not guessed correctly.
if (parseInt(guess) === randomNumber) { correctGuess = true; }
-
The loop checks the while condition - if the condition inside the parentheses matches, it will continue to loop:
while(!correctGuess)
It is important to note here that while(!correctGuess) is shorthand for saying "while correctGuess is false" or "while NOT correctGuess". This is the effect of the exclamation point (!) character - it means "not". Since correctGuess is false as long as the user has not guessed the correct answer, we want it to keep asking the user to guess until they get it right. When they do guess correctly, correctGuess is set to true, and the loop breaks.
I can see how this would be confusing - the while loop continues "when the condition is true", but we're looking for a NOT condition... so we're looking for "correctGuess is false" to be "true". It really just means that whatever is in the parentheses has to be a correct statement for the loop to continue - in the parentheses it basically says "correctGuess is false". So, each time through the loop, it checks to see if that is a correct statement - is correctGuess false? If so, it continues.
Does that make sense?

Tanya Sweeney
14,581 PointsHi Dave,
Do { prompt } while ( correctGuess) is a shorter way of saying do { prompt } while ( correctGuess === true ).
Do { prompt } while ( ! correctGuess ) is a shorter way of saying do { prompt } while ( correctGuess === false).
As long as correctGuess equals false, we have a ( ! correctGuess ) or ( correctGuess === false ) condition, which means the loop will keep going. As soon as correctGuess equals true, we no longer have a ( ! correctGuess ) or ( correctGuess === false ) condition, so we break out of the loop.
I hope that helps!
Tanya

Kris oi
4,910 PointsI get what your saying the loop only runs if the correctGuess is false but the correctGuess was already false but the while condition was set to !correctGuess so that means if correctGuess is true break loop....put pasted in the code below ....if could help would really appreciate it thanks
var randomNumber = getRandomNumber(10); var guess; var guessCount = 0; var correctGuess = false;
function getRandomNumber( upper ) { var num = Math.floor(Math.random() * upper) + 1; console.log(num); return num; } do { guess = prompt('I am thinking of a number between 1 and 10. What is it?'); guessCount+=1; if (parseInt(guess) === randomNumber){ correctGuess = true; } } while(!correctGuess) document.write('<h1> You guessed the number!</h1>'); document.write ('it toke ' + guessCount + ' tries to guss the right number');

Enemuo Felix
1,895 PointsI also have the same confusion as per Kris. So what I just did was put his code in a markdown. Katie Wood Steven Parker Can you guys help explain this better? I can't quite wrap my head around this one
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var guessCount = 0;
function getRandomNumber( upper ) {
return num;
} do
guess = prompt('I am thinking of a number between 1 and 10. What is it?');
guessCount+=1;
if (parseInt(guess) === randomNumber) {
correctGuess = true;
}
} while(!correctGuess)
document.write('<h1> You guessed the number!</h1>');
document.write ('it toke ' + guessCount + ' tries to guss the right number');

Enemuo Felix
1,895 PointsI now understand. You turned up once again!

Thomas Becket
19,820 PointsI understand why ( ! correctGuess ) works. But why does : (correctGuess = false) not work? Shouldn't it actually work as well?
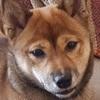
Katie Wood
19,141 PointsHi there,
(correctGuess = false) doesn't work because a single equals sign represents setting a value, not a value comparison. However, if you use == or === it does work. The exclamation point is just shorthand.
Hope this helps!

Thomas Becket
19,820 PointsOhhh... I see thank you for the quick answer!
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsThanks Katie. I understood until I got to N0.3 .If I understand you correctly :
while ( !correctGuess )
In Dave's code Simply means the loop will keep on going until it becomes true. But my confusion is this particular shorthand
!correctGuess
. According to Dave and You this ! means NOT. So when it is added in front of correctGuess it becomes NOT CorrectGuess but Dave already assigned it false in the beginning of the codevar correctGuess = false;
so when it is used in the parenthesis like this
while( !correctGuess)
It is suppose to be NOT False(which is true) No?
Katie Wood
19,141 PointsKatie Wood
19,141 Pointswhile (!correctGuess) means "while correctGuess is false" - the value the variable is initialized with doesn't change the meaning. He sets it to that value at the beginning to ensure that the loop will continue until correctGuess is set to true. Using only a variable's name in a conditional is a form of shorthand as well - for example, these two are the same:
Likewise, these are the same:
Does that clear things up?