Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial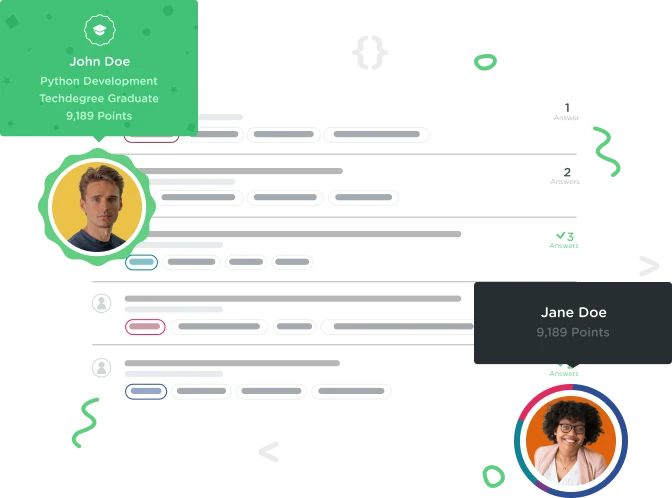
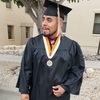
Julian Cardona
Python Web Development Techdegree Student 4,894 PointsNot responding
I'm having trouble solving the challenge. class Letter: def init(self, pattern=None): self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, output):
sep = []
new_out = []
for i in output:
together = i.split('-')
for i in together:
if i == 'dot':
new_out.append('.')
else:
new_out.append('_')
return cls(new_out)
class S(Letter): def init(self): pattern = ['.', '.', '.'] super().init(pattern)
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls,pattern):
pattern = Letter(pattern)
return cls(pattern)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
2 Answers
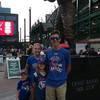
AJ Salmon
5,675 PointsHey Julian,
The first for loop in your class method is unnecessary. Get rid of the loop, and then assign output.split('-') to the variable together, and it should pass!
@classmethod
def from_string(cls, output):
#you also had an empty list "sep" here, that wasn't needed either!
new_out = []
together = output.split('-')
for i in together:
if i == 'dot':
new_out.append('.')
else:
new_out.append('_')
return cls(new_out)
Hope this helps, and happy coding!
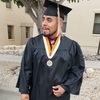
Julian Cardona
Python Web Development Techdegree Student 4,894 PointsThe reason i used the first loop was that the argument output is a list, which i want to rid of '-'.
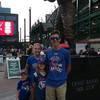
AJ Salmon
5,675 PointsYes, but you can do this in one step! Your loop is looping through the initial argument, but the argument is a string, so you can can simply .split() it on the hyphen once. There's no need to loop through it. Example:
>>> output = 'dash-dot-dot-dash-dash'
>>> together = output.split('-')
>>> together
['dash', 'dot', 'dot', 'dash', 'dash']
Splitting the string on the hyphen creates the list that you need, without looping through it :)
Julian Cardona
Python Web Development Techdegree Student 4,894 PointsJulian Cardona
Python Web Development Techdegree Student 4,894 PointsAJ Salmon This is the challenge: Let's practice using @classmethod! Create a class method in Letter named from_string that takes a string like "dash-dot" and creates an instance with the correct pattern (['_', '.']).