Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial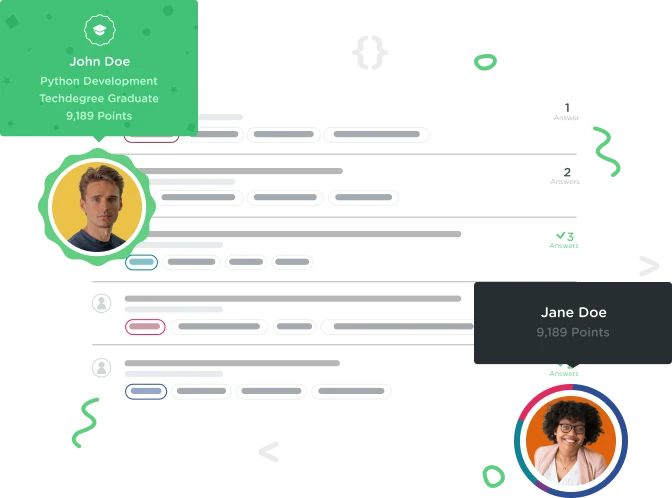
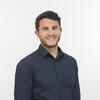
Isaac Arnold
Front End Web Development Techdegree Graduate 15,863 PointsNot returning a result
For some reason my console isn't returning a value...any ideas why? Here is my code:
function calculate_gpa(student_grades) {
let grade_total = 0;
let num_of_grades = student_grades.length;
for (let i = 0; i < num_of_grades.length; i++) {
let grade = student_grades[i];
if (grade < 1 || grade > 4) {
console.log(`Invalid grade: ${grade}`);
return "Can't calculate grade.";
} else {
grade_total += student_grades[i];
}
}
const gpa = grade_total / num_of_grades;
return gpa;
}
let reggie_grades = [4, 4, 3, 4];
console.log(calculate_gpa(reggie_grades));
2 Answers
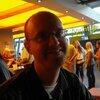
Robert Gulley
Front End Web Development Techdegree Student 10,722 PointsHi Isaac! -
You're very close! The problem is in the initial line of your for statement. You need to set your condition to evaluate the length of your array. Since you are passing the array to the student_grades argument, you want to test the .length of student_grades, like so:
for (let i = 0; i < student_grades.length; i++) {
When you assigned this value to num_of_grades, the length (in this case 4), was assigned, and 4 is not an array, so when you called num_of_grades.length in your for statement, the length was 0 and your code never went through the for loop because 0 is not less than 0.
Hope this helps!
Mod Edit: Changed comment to answer so it may be voted on or marked as best. Thank you!
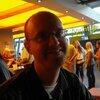
Robert Gulley
Front End Web Development Techdegree Student 10,722 PointsIsaac -
When I run the code you just posted, I get the correct answer of 3.75. Are you saving your file and reloading the browser?
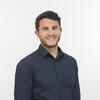
Isaac Arnold
Front End Web Development Techdegree Graduate 15,863 PointsHey Robert - for some reason I don't think I was running node gpa.js in the correct directory so it was returning 0. I've managed to get it to return the correct result now. Thanks so much for your advice and patience, greatly appreciated!
Isaac Arnold
Front End Web Development Techdegree Graduate 15,863 PointsIsaac Arnold
Front End Web Development Techdegree Graduate 15,863 PointsHey Robert! Thanks for reviewing my question and supplying a super helpful and comprehensive answer. I've changed my for loop statement, but it seems to still be returning 0. I'm not sure what I'm doing wrong here as everything seems to be the same as what Dave explained in the video...? Here is my code now: