Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial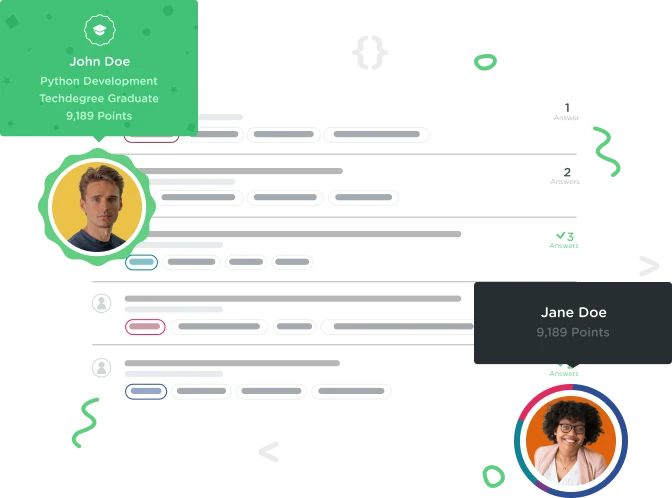
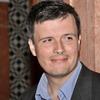
Brian Patterson
19,588 PointsNot returning any data from Ajax request.
I am trying to get some practice in sending requests to API's. So I thought I would try a random API to sharpen my skills. I am using JQuery and AJAX to access a formula one API. Here is my code so far.
$(document).ready(function(){
$('#submitYear').click(function(){
let year = $("#year").val();
if(year != ''){
//Get the Ajax request
$.ajax({
url:"http://ergast.com/api/f1/" + year + "/circuits.json?callback=par",
type: "GET",
dataType: "json",
success: function(data){
let widget = show(data);
$("#show").html(widget);
$("#year").val('');
}
});
}else {
$("#error").html('Field cannot be empty');
}
});
});
function show(data) {
let circuitHtml = '<ul>';
$.each(data.Circuits, function(i, place){
circuitHtml += '<li> name: '+ place.circuitName + '</li>';
});
circuitHtml += '</ul>';
}
The problem I am having is that it is not returning anything to html page. Here is my html.
<!doctype html>
<html lang="en">
<head>
<title>The Circuits at Which Formula One Season</title>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta.2/css/bootstrap.min.css" integrity="sha384-PsH8R72JQ3SOdhVi3uxftmaW6Vc51MKb0q5P2rRUpPvrszuE4W1povHYgTpBfshb" crossorigin="anonymous">
</head>
<body>
<div class="jumbotron" style="margin-bottom:0px color:white; background-color: #4aa1f3;">
<h2 class="text-center text" style="font-size:50px; font-weight:600;">Enter a year of circuits</h2>
</div>
<div class="container">
<div class="row" style="margin-bottom:20px;">
<h3 class="text-center text-primary">Enter Year</h3>
<span id="error"></span>
</div>
<div class="row form-group form-inline justify-content-center" id="rowDiv">
<input type="text" name="year" id="year" class="form-control" placeholder="Year">
<button id="submitYear" class="btn btn-primary">Seach Year</button>
</div>
<div id="show"></div>
</div>
<!-- Optional JavaScript -->
<!-- jQuery first, then Popper.js, then Bootstrap JS -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.3/umd/popper.min.js" integrity="sha384-vFJXuSJphROIrBnz7yo7oB41mKfc8JzQZiCq4NCceLEaO4IHwicKwpJf9c9IpFgh" crossorigin="anonymous"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta.2/js/bootstrap.min.js" integrity="sha384-alpBpkh1PFOepccYVYDB4do5UnbKysX5WZXm3XxPqe5iKTfUKjNkCk9SaVuEZflJ" crossorigin="anonymous"></script>
<script src="js/app.js"></script>
<!-- Latest compiled and minified JavaScript -->
</body>
</html>
Any ideas on how I can fix this would be appreciated.
1 Answer
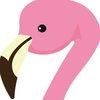
Dave StSomeWhere
19,870 PointsVery cool test project. If you debug your script (in something like firebug or firebug lite) you'll be able to work through your issues.
With the below fixes and running it for 2015 I get the following:
- name: Albert Park Grand Prix Circuit
- name: Circuit of the Americas
- name: Bahrain International Circuit
- name: Circuit de Barcelona-Catalunya
- name: Hungaroring
- name: Autódromo José Carlos Pace
- name: Marina Bay Street Circuit
Issues list:
HTML - missing semi-colon after 0px in jumbotron div margin bottom
JavaScript: In the URL you specify a callback called "par" which is not defined - I just removed it
In the function show you never return anything
In the returned data (which you can see in debug) you need to specify - data.MRData.CircuitTable.Circuits for the .each
FYI - console.log() is your best buddy
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsSorry if this is a silly question in the show function do I return with ?
Thanks for your help Dave.
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsPlease see below Dave.