Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial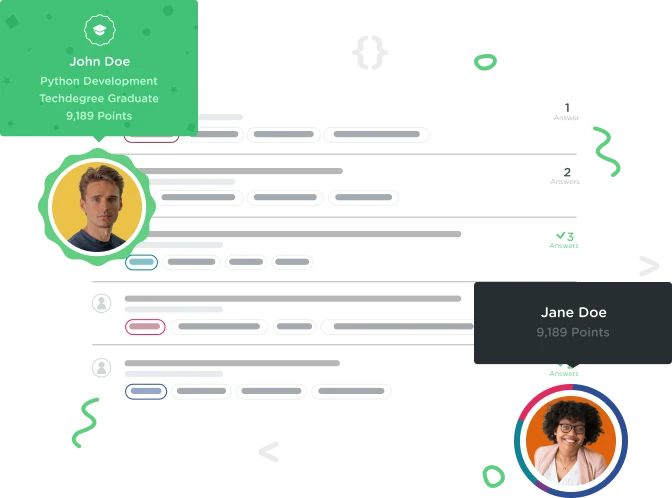

Jazz Atkin
2,869 PointsNot returning student data, don't know why
Can anyone explain why this isn't returning any data when a correct name is entered?
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: 175,
points: 16375
},
{
name: 'John',
track: 'PHP Development',
achievements: 55,
points: 2025
},
{
name: 'John',
track: 'Learn WordPress',
achievements: 40,
points: 1950
},
{
name: 'Trish',
track: 'Rails Development',
achievements: 5,
points: 350
}
];
var html;
var student;
var input;
// print message within output div
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// return student report as ordered list
function getReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<ol><li>Track: ' + student.track + '</li>';
report += '<li>Points: ' + student.points + '</li>';
report += '<li>Achievements: ' + student.achievements + '</li></ol>';
return report;
}
// prompt user for student name
while (true) {
input = prompt('Enter a students name, or type quit to exit.');
// break loop if user inputs quit
if (input === null || input.toLowerCase() === 'quit') {
break;
}
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
if ( student.name === input ) {
html += getReport(student);
print(html);
}
}
}
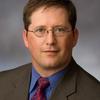
Ted Sumner
Courses Plus Student 17,967 PointsHmmm. What I was working to reformat is different than what is posted now.
4 Answers

Iain Diamond
29,379 PointsI've added a link to a working workspace snapshot below. I changed a couple of lines to get it to work nicely
var html = ""; // line 1
if (input !== null && input.toLowerCase() === 'quit') { // line 24
You need to initialise the html string, otherwise it prints out undefined to the browser window. This makes sense as the variable is never initialised, so is actually undefined.
There was a console error, which I fixed by adding an extra check on line 24.
iain
p.s. to run the snapshot you need to fork it, apparently.

Jazz Atkin
2,869 PointsThank you!!

Iain Diamond
29,379 PointsYou're looping over a students array. Where is that setup?

Jazz Atkin
2,869 PointsIt was stored in a separate js, I've added it into my code here now for clarity!
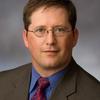
Ted Sumner
Courses Plus Student 17,967 PointsHere is the error I get in the JavaScript console:
Uncaught TypeError: Cannot set property 'innerHTML' of null at print (<anonymous>:42:23) at <anonymous>:65:13 at Object.InjectedScript._evaluateOn (<anonymous>:895:140) at Object.InjectedScript._evaluateAndWrap (<anonymous>:828:34) at Object.InjectedScript.evaluate (<anonymous>:694:21)
Unfortunately, I don't remember enough about JavaScript to give you help beyond that. I am going to have to retake the course.

Jazz Atkin
2,869 PointsI have amended the issue causing that response in the console - it relates to a user cancelling the prompt window, but I'm still unable to get anything to print when I enter a name that does exist in the array.
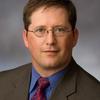
Ted Sumner
Courses Plus Student 17,967 PointsI got that error when I added Dave to the user input window. Also, please repost code when you make changes so we can see what is different.
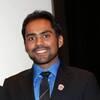
Ismail Qaznili
27,996 PointsThe code is perfect!
All you have to do is write the name in a case-sensitive manner; such as 'Dave' rather than 'dave'.
Perhaps we need to add .toLowerCase() to input and student.name to avoid any bugs.
Let me know if it works for you too.
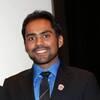
Ismail Qaznili
27,996 PointsI also noticed that if you started to cycle through the names, the html variable retains all the previous information. No idea how that can be treated.
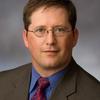
Ted Sumner
Courses Plus Student 17,967 PointsHe is taking the variable and concatenating new information to it. It is the same as report = report + '<li>Points: ' + student.points + '</li>;
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 PointsYou almost have the markdown quote correct. After the three ` add the programming language, then start the quote on the next line. Put the three closing ` on the line after the quote. The ` is on the upper left of your keyboard.