Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial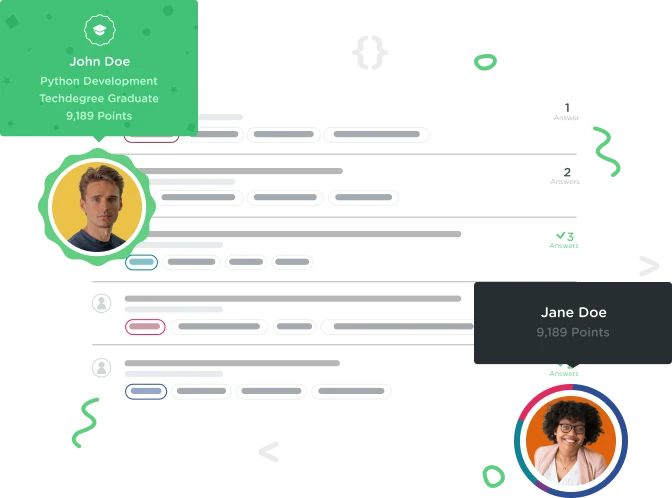

Denis Saidov
Courses Plus Student 91 PointsNot showing the log.
It is simply not showing the console.log after clicking the button. The variables seem to be set correctly to me. Don't get where I am wrong.
Here is the .js file:
var emailInput = document.getElementsByName("email"); // the email input value
var passwordInput = document.getElementsByName("password"); // the password input value
var submitButton = document.getElementsByTagName("button"); // the actual submit button
var emptyCheck = function(){
console.log("Checking for empty inputs...");
}
submitButton.onclick = emptyCheck;
This is the .html file:
<form action="javascript:;" method="post" name="login_form" class="login-form">
<h1>Login</h1>
<div class="field">
<input id="email" placeholder="Email address" type="text" name="email">
<p id="error-message" class="error-message"><span>Test</span></p>
</div>
<div class="field">
<input id="password" placeholder="Password" type="password" name="password">
</div>
<div class="row">
<div class="col-6 col-lg-6 col-sm-12">
<button id="button" class="button" name="submitButton">Login</button>
</div>
<div class="col-6 col-lg-6 col-sm-12">
<div class="note"><div class="title">Having trouble logging in?</div>
<a href="/lost-password/index.php" target="_self">Reset my password</a>
</div>
</div>
</div>
</form>
When I open the page and type submitButton at the console it is not showing me "Checking for empty inputs..."
3 Answers

Nega Lulessa
15,669 PointsDenis,
May i suggest you use the ID selector instead. You can't go wrong with selecting an ID on any given page since it is unique to that page. Any traversal you do to gather up the element with that ID will only return (if that ID exists on that page) a single element with that unique ID.
Hence, in your JavaScript code:
change this:
var submitButton = document.getElementsByTagName("button");
to this:
var submitButton = document.getElementById("button");
I tried it in my browser's console (chrome) and it worked.
By the way, Hugo's method of picking the returned array apart also works. I prefer the traversal through the element's ID since that returns ONE element as opposed to an array that requires further fine-tuning.
Do shout back should you still have questions on this issue...
Cheers!
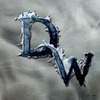
Hugo Paz
15,622 PointsHi Denis,
Change this:
var submitButton = document.getElementsByTagName("button");
To this:
var submitButton = document.getElementsByTagName("button")[0];
The method getElementsByTagName returns an array of HTML elements, in this case buttons. Since there is only one on the page, you need to add the index 0 to get that button.
I have tried this and the message appears on the console but only briefly.

Denis Saidov
Courses Plus Student 91 Points
As you can see the function and the variable are linked correctly. However, when I click the button, it is not showing me "Checking for empty inputs...". The button is not working, which is strange.
Just in case, I am giving you the CSS file related to this, but it seems that the problem is elsewhere:
.button {
display: block;
width: 100%;
margin-bottom: 10px;
font-size: 18px;
font-weight: 300;
}
I am really sorry for bothering you guys, but I really don't see where I have made a mistake. Thanks in advance.
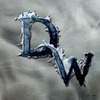
Hugo Paz
15,622 PointsDenis like i said what is stored in the submitButton variable is not the button, but an array of buttons.
If you look at your image you can see that the button html is surrounded by square brackets.
You need to explicitly tell which button you want to trigger the function.
By changing your code from:
var submitButton = document.getElementsByTagName("button");
to
var submitButton = document.getElementsByTagName("button")[0];
it works as you intended.
Another alternative is to select the element in the array when you call the function, like this:
submitButton[0].onclick = emptyCheck;

Jane Alexander
1,979 PointsTry reloading the page