Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial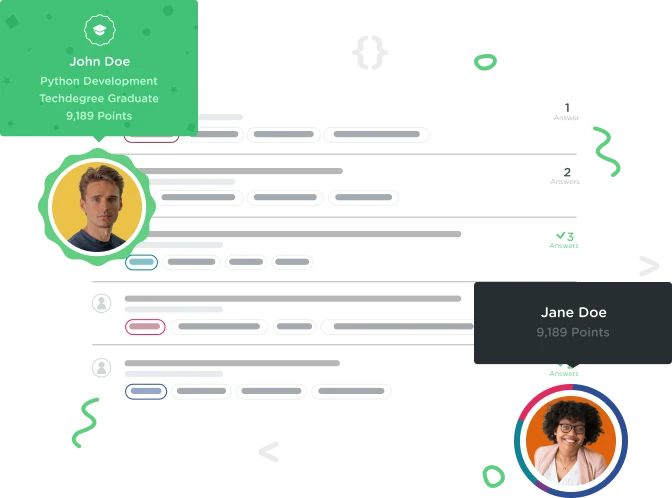

Yashu Jain
518 PointsNot Showing Time
This is my code for controller Home.java:
package com.teamtreehouse.pomodoro.controllers;
import com.teamtreehouse.pomodoro.Attempt; import com.teamtreehouse.pomodoro.AttemptKind; import javafx.animation.KeyFrame; import javafx.animation.Timeline; import javafx.beans.property.SimpleStringProperty; import javafx.beans.property.StringProperty; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.util.Duration;
/**
-
Created by rushabhajain on 10/01/2017. */ public class Home { @FXML private Label title; @FXML private VBox container;
private Attempt mCurrentAttempt;
private StringProperty mTimerText;
private Timeline mTimeline;
public Home(){ mTimerText = new SimpleStringProperty(); setmTimerText(0); }
public String getmTimerText() { return mTimerText.get(); }
public StringProperty mTimerTextProperty() { return mTimerText; }
public void setmTimerText(String mTimerText) { this.mTimerText.set(mTimerText); }
public void setmTimerText(int remainingSeconds) { int minutes = remainingSeconds / 60; int seconds = remainingSeconds % 60; setmTimerText(String.format("%02d:%02d",minutes,seconds)); }
private void prepareAttempt(AttemptKind kind){ clearAttemptStyles(); mCurrentAttempt = new Attempt(kind, ""); addAttemptStyle(kind); title.setText(kind.getmDisplayName()); }
private void addAttemptStyle(AttemptKind kind){ container.getStyleClass().add(kind.toString().toLowerCase()); }
private void clearAttemptStyles(){ for (AttemptKind kind : AttemptKind.values()){ container.getStyleClass().remove(kind.toString().toLowerCase()); } }
public void DEBUG(ActionEvent actionEvent) { System.out.println("HI MOM"); }
}
This is my code for home.fxml: <?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.?> <?import javafx.scene.image.Image?> <?import javafx.scene.image.ImageView?> <?import javafx.scene.layout.?> <?import javafx.scene.shape.SVGPath?> <VBox stylesheets="/css/main.css" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml" fx:controller="com.teamtreehouse.pomodoro.controllers.Home" fx:id="container"> <children> <Button fx:id="DEBUG" onAction="#DEBUG" > Debug </Button> <Label fx:id="title" text="Pomodoro"/>
<Label fx:id="time" text="${controller.mTimerText}"/>
<HBox styleClass="buttons">
<children>
<StackPane>
<children>
<StackPane styleClass="nested-action,play">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M1.6 17C1 17 0 16.2 0 15.6V1.3C0 .1 2.2-.4 3.1.4l8.2 6.4c.5.4.8 1 .8 1.7 0 .6-.3 1.2-.8 1.7l-8.2 6.4c-.5.2-1 .4-1.5.4z"/>
</HBox>
<Button text="Resume"/>
</children>
</StackPane>
<StackPane styleClass="nested-action,pause">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M10.2 17c-1 0-1.8-.8-1.8-1.8V1.8c0-1 .8-1.8 1.8-1.8S12 .8 12 1.8v13.4c0 1-.8 1.8-1.8 1.8z" />
<SVGPath styleClass="svg"
content="M10.2 17c-1 0-1.8-.8-1.8-1.8V1.8c0-1 .8-1.8 1.8-1.8S12 .8 12 1.8v13.4c0 1-.8 1.8-1.8 1.8z" />
</HBox>
<Button text="Pause"/>
</children>
</StackPane>
</children>
</StackPane>
<StackPane styleClass="nested-action,restart">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M21 2.9C19.1 1 16.6 0 13.9 0c-1.1 0-2 .9-2 1.9s.9 1.9 2 1.9c1.7 0 3.2.6 4.4 1.8 2.4 2.4 2.4 6.3 0 8.7-1.2 1.2-2.7 1.8-4.4 1.8-1.7 0-3.2-.6-4.4-1.8-1.8-1.8-2.3-4.4-1.4-6.7L9.3 10c.2.4.5.7.9.8.4.1.8.1 1.2-.1.8-.4 1.1-1.3.8-2.1L10 4.3c0-.5-.2-1-.6-1.4l-.1-.1-.1-.2c-.2-.4-.5-.7-.9-.8-.4-.1-.8-.1-1.2.1L.9 4.8C.1 5.2-.2 6.1.2 6.9c.2.4.5.7.9.8.4.1.8.1 1.2-.1l2-.9c-1.2 3.5-.4 7.6 2.4 10.4C8.6 19 11.2 20 13.9 20s5.3-1 7.2-2.9C25 13.2 25 6.8 21 2.9z"/>
</HBox>
<Button text="Restart"/>
</children>
</StackPane>
</children>
</HBox>
<TextArea fx:id="message" promptText="What are you doing?"/>
<ImageView>
<image>
<Image url="/images/pomodoro.png"/>
</image>
</ImageView>
</children>
</VBox>
2 Answers
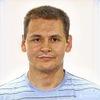
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsLooks like you have problem with getters/setters, i.e. when you write
<Label fx:id="time" text="${controller.mTimerText}"/>
Program is looking for methods setTimerText
and getTimerText
Inferring from your code
// wrong ! should be "getTimerText"
public String getmTimerText() {
return mTimerText.get();
}
// wrong ! should be "setTimerText"
public void setmTimerText(String mTimerText) {
this.mTimerText.set(mTimerText);
}
// wrong should be "setTimerText"
public void setmTimerText(int remainingSeconds) { int minutes = remainingSeconds / 60; int seconds = remainingSeconds % 60; setmTimerText(String.format("%02d:%02d",minutes,seconds)); }
There will be probably MORE errors, so you have to actually go through all your code and change things.
The best way I suggest is go to Downloads section of the video on which you are in right now and download right files And compare them with yours. They should work 100 %.
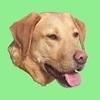
alastair cooper
30,617 PointsThank you Alexander for this answer. I had the same problem and had no idea what to do until I read this.
For anyone else getting here, it is not too bad to solve. I simply (refactored / renamed) mTimerText to timerText. The IDE updated all the getters and setters for me and the code ran straight away