Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial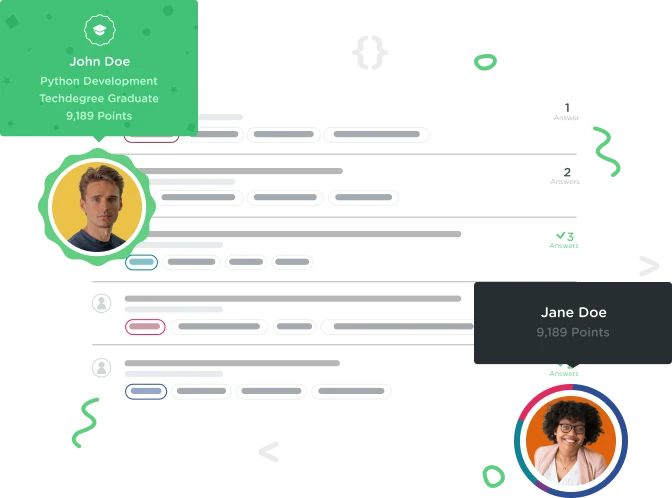
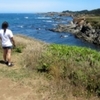
Nancy Melucci
Courses Plus Student 35,157 PointsNot sure how to code this function
I am supposed to create a function to log movements for this game, deduct points for hitting a wall and keep the player from moving past the wall.
Since I don't have any other game functions or variables (and I don't have much experience with game programming) I can't really get past this point. I know how to deduct the points for hitting the wall (I know the code is not DRY but it is mathematically correct) but since I have so little other information about the game I can't figure out how to finish the function and have it do all these things.
Would appreciate a hint or some additional code if it exists.
Thanks.
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction):
x, y, hp = player
if x == 0:
hp = hp - 5 and x == 0
elif x == 9:
hp = hp - 5 and x == 9
elif y == 0:
hp = hp - 5 and y == 0
elif y == 9:
hp = hp - 5 and y == 9
return x, y, hp
6 Answers

Umesh Ravji
42,386 Pointsx, y, hp = player
This is where the player currently is, the x and y locations obtained here. These need to be updated before you begin the checks to see if the player has moved beyond the walls.
x += direction[0] # update player x
y += direction[1] # update player y
In order to update the x position, you use the first item from the tuple, and update the y position using the secound item from the tuple.

Umesh Ravji
42,386 PointsYour player is at x, y to begin with, you first have to use the direction tuple to modify the players x and y position.
Once the position has been updated, you can then check to see if the player has left the bounds. I have changed the numbers, but there's a few different ways you could check this. I am not sure why you have used a comparison here:
hp = hp - 5 and x == 0
Also remember that you can if else the x coordinates, and y coordinates.. but not together because a player may move off the game area diagonally. Hope this helps :)
def move(player, direction):
x, y, hp = player
# update position here
if x == -1:
hp = hp - 5
x = 0
elif x == 10:
hp = hp - 5
x = 9
if y == -1:
hp = hp - 5
y = 0
elif y == 10:
hp = hp - 5
y = 9
return x, y, hp
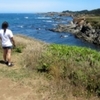
Nancy Melucci
Courses Plus Student 35,157 PointsYes, that makes sense. But I am also having a hard time figuring out how to include the code for the player's move in the function. Probably because of my lack of experience, the function being un-connected to any aspect of the game makes it hard for me to do that. Would it be something like this?
I am really in the weeds.
x, y, hp = player
direction = x, y
if x == -1:
hp = hp - 5
x = 0
elif x == 10:
hp = hp - 5
x = 9
if y == -1:
hp = hp - 5
y = 0
elif y == 10:
hp = hp - 5
y = 9
return x, y, hp
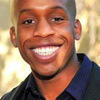
Max Madubuike
Full Stack JavaScript Techdegree Student 8,258 PointsI won't post the correct answer, however, after successfully completing the challenge, I will post some hints.
First, the move function we are given must assign the value in 2 tuples. The first is the player tuple which states the players' current position and hp. The function unpacks this tuple with x, y, hp = player.
The second tuple that needs to be unpacked is the movement tuple. This is left for you to assign.
After that, it is very helpful to define the bounds of failure for this challenge. That is, "Where does the player lose HP?" In this challenge, the player will lose HP if they attempt to leave the bounds of the board. That is defined as the corners, and the 4 walls/edges.
You can use a series of condition statements to test the players current position and ensure they remain in the board as well as lose HP. I've done one of them for you. The rest I will leave to you.
# bottom left corner
if (x == 0 and y == 0) and (xm or ym == -1):
x = 0
y = 0
hp -= 5
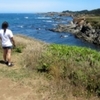
Nancy Melucci
Courses Plus Student 35,157 PointsI see also what you say about updating the tuple. Do I need to unzip it somehow? Tuples are immutable, so that is also puzzling to me. The code above doesn't pass. It just represents another attempt by me.
Thanks.
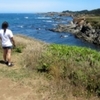
Nancy Melucci
Courses Plus Student 35,157 PointsThank you for the generous assist.
NJM

saidakhmedbayev
2,210 PointsHere is what I have done:
if x == 0 or x == 9:
if x == 0 and dir_x == -1:
hp -= 5
x = x
elif x == 9 and dir_x == 1:
hp -= 5
x = x
elif dir_x == -1:
x -= 1
elif dir_x == 0:
x = x
else:
x += 1