Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial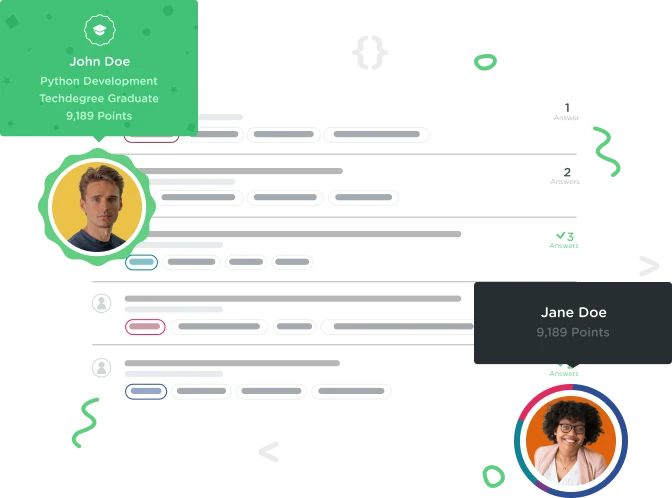
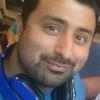
Parvinder Grewal
3,678 PointsNot sure how to compare list items with dictionary keys.
def members(dictArg, keysListArg):
count = 0
for x in my_list:
if my_list[x] == my_dict.keys():
count += 1
return count
#I am not sure how to iterate through a list and than compare it with the dictionary keys.
[MOD: added ```python markdown formatting -cf]
2 Answers
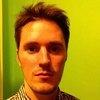
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThe .keys() method on a dictionary is going to return a list. You can't just compare that whole list to each item in the other list, you have to compare item to item. Also, you have to use the parameter names that you defined, dictArg and keysListArg, not my_list and my_dict. Those might be the names of actual things you pass in, but within the function you need to stay consistent. (You could also just call your parameters my_list and my_dict).
Here's how I solved it. There's a loop within a loop. For each list_value, it's going to loop through each dict_value and compare them, and then do it all over again for the next list_value.
def members(dictArg, keysListArg):
count = 0
for list_item in keysListArg:
for dict_key in dictArg.keys():
if list_item == dict_key:
count+= 1
return count
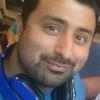
Parvinder Grewal
3,678 PointsBrendan Whiting: Thank you for your response, excuse my typo with the parameter names.
Chris Freeman Thank for your help once again. I'm glad my answer was somewhere close.
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsThis works but can be improved: You don't need to check each
list_item
individually against eachdictArg
key. Instead you can use thein
keyword. Also, when referring to a dictionary as an iterable, operating over the keys is assumed, so the.keys()
is unnecessary:Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsWish I could upvote your answer.
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsNo worries. Since my derivative work was based on yours, I wanted to extend your answer so that you would get points when this gets "Best Answer". Everybody wins!