Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial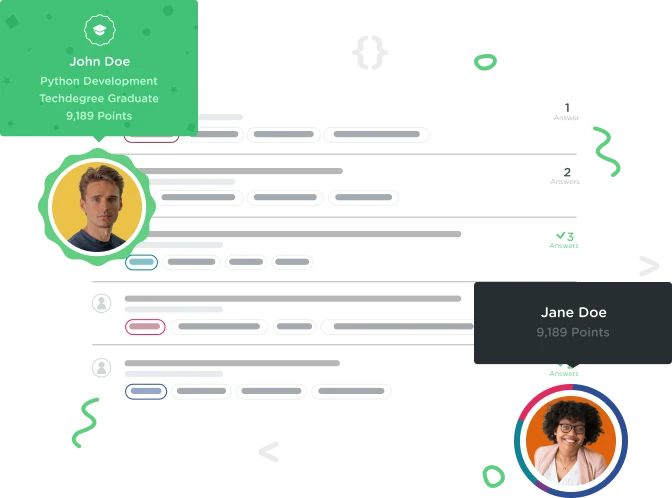
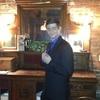
Carl Smith
8,185 PointsNot sure how to create and instance and assign to someShape
Confused on what this means.
// Enter your code below
class Shape{
var numberOfSides: Int
init(numberOfSides: Int){
self.numberOfSides = someShape
}
}
2 Answers
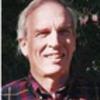
jcorum
71,830 PointsThere was a slight problem in your initializer:
class Shape{
var numberOfSides: Int
init(numberOfSides: Int){
self.numberOfSides = numberOfSides
}
}
let someShape = Shape(numberOfSides: 6)
When the initializer is called you will send it an int (say 6). The initializer takes that value and assigns it to its stored property with this statement: self.numberOfSides = numberOfSides
What it's saying is: assign to my stored property named numberOfSides (self.numberOfSides) the value passed in by the parameter numberOfSides.
Then, once the class is fixed up, you can create a Shape object named someShape by calling the initializer with an int value. Don't forget that you have to provide the name of the parameter as well as the value.
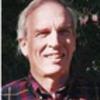
jcorum
71,830 PointsThe purpose of the initializer is to (1) create an object from the class, and (2) before doing so to make sure every stored property of the class has a value before the object is created. In this case it is to make sure every Shape object has its number of sides specified. Or, in other words, to make sure the stored property numberOfSides has an int value.
Since the initializer takes an int you cannot do this:
let someShape = Shape()
or this:
let someShape = Shape(numberOfSides: "3")
If the Shape class had two stored properties: numberOfSides and color, then the constructor would look like this:
``swift init(numberOfSides: Int, color: String){ self.numberOfSides = numberOfSides self.color = color }
and you would create Shape objects like this:
```swift
let someShape = Shape(numberOfSides: 6, color: "red")
Carl Smith
8,185 PointsCarl Smith
8,185 PointsI'm still a little foggy on the initializer, I know how to write it, just not sure what it's purpose is. I'll watch the video again and hopefully get more familiar with it.