Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial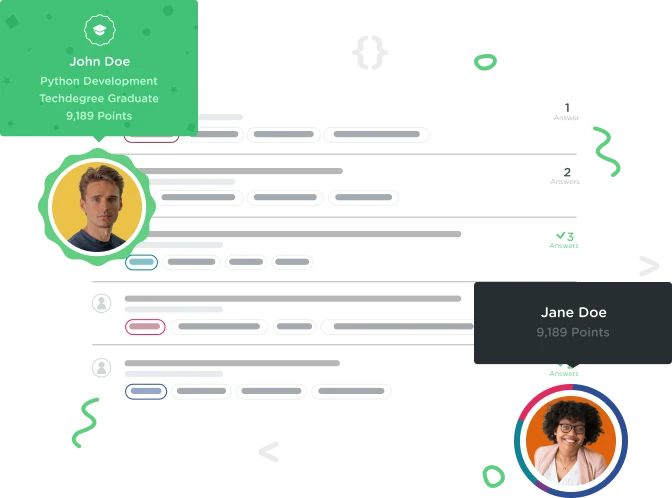
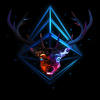
Anthony Ramnarain
8,951 PointsNot sure how to fix error message: Attempt to invoke virtual method on a null reference
2018-11-16 18:37:40.649 14685-14685/com.anthonyramnarain.interactivestory E/sdcard-err2:: Attempt to invoke virtual method 'int com.anthonyramnarain.interactivestory.model.Page.getImageId()' on a null object reference
Code:
'package com.anthonyramnarain.interactivestory.model;
public class Page { private int imageId; private int textId; private Choice choice1; private Choice choice2; private boolean isFinalPage = false;
public Page(int imageId, int textId) {
this.imageId = imageId;
this.textId = textId;
this.isFinalPage = true;
}
public Page(int imageId, int textId, Choice choice1, Choice choice2) {
this.imageId = imageId;
this.textId = textId;
this.choice1 = choice1;
this.choice2 = choice2;
}
public boolean isFinalPage() {
return isFinalPage;
}
public void setFinalPage(boolean finalPage) {
isFinalPage = finalPage;
}
public int getImageId(){
return imageId;
}
public void setImageId(int imageId) {
this.imageId = imageId;
}
public int getTextId() {
return textId;
}
public void setTextId(int textId) {
this.textId = textId;
}
public Choice getChoice1() {
return choice1;
}
public void setChoice1(Choice choice1) {
this.choice1 = choice1;
}
public Choice getChoice2() {
return choice2;
}
public void setChoice2(Choice choice2) {
this.choice2 = choice2;
}
}'
This is where I am calling the method:
'package com.anthonyramnarain.interactivestory.ui;
import android.content.Intent; import android.graphics.drawable.Drawable; import android.support.v4.content.ContextCompat; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView;
import com.anthonyramnarain.interactivestory.R; import com.anthonyramnarain.interactivestory.model.Page; import com.anthonyramnarain.interactivestory.model.Story;
public class StoryActivity extends AppCompatActivity {
private String name; private Story story; private ImageView storyImageView; private TextView storyTextView; private Button choice1Button; private Button choice2Button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
storyImageView = (ImageView) findViewById(R.id.storyImageView);
storyTextView = (TextView)findViewById(R.id.storyTextView);
choice1Button = (Button)findViewById(R.id.choice1);
choice2Button = (Button)findViewById(R.id.choice2);
Intent intent = getIntent();
name = intent.getStringExtra(getString(R.string.key_name));
if (name == null || name.isEmpty()) {
name = "Friend";
}
story = new Story();
loadPage(0);
}
private void loadPage(int pageNumber) {
final Page page = story.getPage(pageNumber);
Drawable image =ContextCompat.getDrawable(this, page.getImageId());
storyImageView.setImageDrawable(image);
String pageText = getString(page.getTextId());
// Add name if placeholder included. Won't add if not
pageText = String.format(pageText, name);
storyTextView.setText(pageText);
if (page.isFinalPage()){
choice1Button.setVisibility(View.INVISIBLE);
choice2Button.setText(getString(R.string.play_again_button_text));
}else {
loadButtons(page);
}
}
private void loadButtons(final Page page) {
choice1Button.setText(page.getChoice1().getTextId());
choice1Button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int nextPage = page.getChoice1().getNextPage();
loadPage(nextPage);
}
});
choice2Button.setText(page.getChoice2().getTextId());
choice2Button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int nextPage = page.getChoice2().getNextPage();
loadPage(nextPage);
}
});
}
}'
2 Answers

Lauren Moineau
9,483 Points(Re-posting my above comment as a proper answer)
I had the same problem and it was because page[1] was missing from the constructor in the Story class. I added the following:
pages[1] = new Page(R.drawable.page1,
R.string.page1,
new Choice(R.string.page1_choice1, 3),
new Choice(R.string.page1_choice2, 4));
Just make sure all the pages are there. Hope this helps.
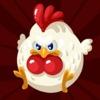
Binyamin Friedman
14,615 PointsIt seems that story.getPage(0)
is returning a null reference. You haven't given us the code for the story class, so I wouldn't know why it is returning a null value. Perhaps you have the same error as Elle M but are missing the first page.
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsI had the same problem and it was because page[1] was missing from the constructor in the Story class. I added the following:
Just make sure all the pages are there. Hope this helps.