Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial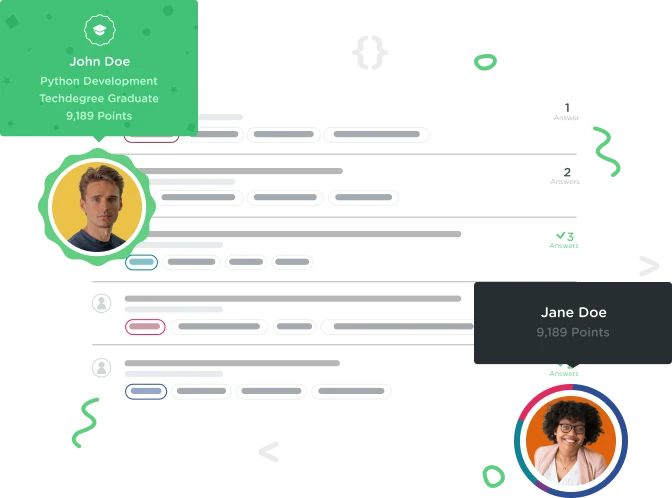

Pierce Mulligan
1,106 PointsNot sure how to set the bar count to 0 on the drive method.
Where is this program failing?
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
if{mBarsCount =< 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
1 Answer
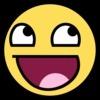
Michael Hess
24,512 PointsHi Pierce,
In this problem it wants us to throw an illegal argument exception if the number of laps exceeds the battery charge level. The illegal argument exception should read "Not enough battery remains"
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if (laps > mBarsCount){
throw new IllegalArgumentException ("Not enough battery remains");
}
mBarsCount -= laps;
}
We can accomplish this by making an if-statement that tests if the laps exceeds the number of battery bars. If the laps exceeds the bars count an illegal argument exception is thrown.
If the bars count exceeds the number of laps, mbarsCount is decremented by 1 lap.
The finished product should look like:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if (laps > mBarsCount){
throw new IllegalArgumentException ("Not enough battery remains");
}
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
If you have any other questions go ahead and shout them out! Hope this helps!