Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial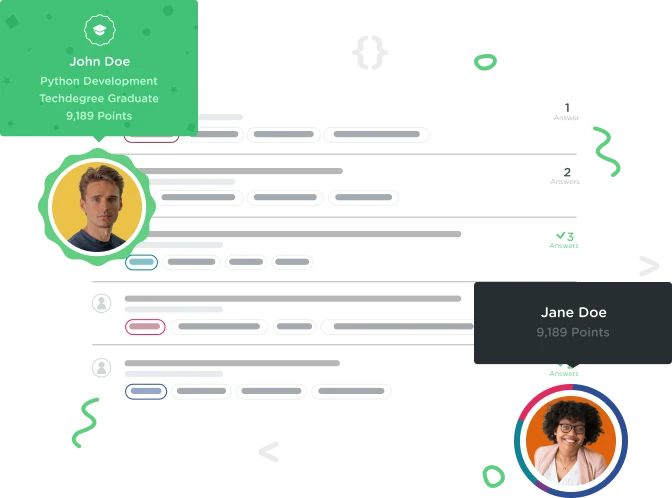
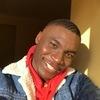
James Nunoo
3,668 Pointsnot sure I understand this question on arrays
need a little help
var temperatures = [100,90,99,80,70,65,30,10];
function new(num){
for (var i = 100; i < temperatures.length i += 1){
console.log(num);
}
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
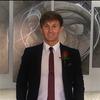
Liam Clarke
19,938 PointsHi James
No need for the function, you just need to loop through each of the array items getting the array index value.
Without a loop this would look something like this:
var temperatures = [100,90,99,80,70,65,30,10];
console.log(temperatures[0]);
console.log(temperatures[1]);
console.log(temperatures[2]);
console.log(temperatures[3]);
// etc
However, the question is to do that in a loop with either a for loop or while loop. This can be done by looping over the array length and console logging each iteration, like below:
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
}
Alternatively, using a while loop, it would look like this:
var temperatures = [100,90,99,80,70,65,30,10];
var i = 0;
while (i < temperatures.length) {
console.log(temperatures[i]);
i++;
}
Notice that each of the examples i provide follow a similar pattern:
- a variable is set to iterate over
i
- a conditional is made to check if the iteration variable is less that the array length
i < temeratures.length
- After each console log, the iteration variable is incremented by 1
i++
Both of these loops are identical in there output and both do the exact same thing, but are syntactically different.
Hope this helps!
James Nunoo
3,668 PointsJames Nunoo
3,668 PointsThank you Liam!