Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial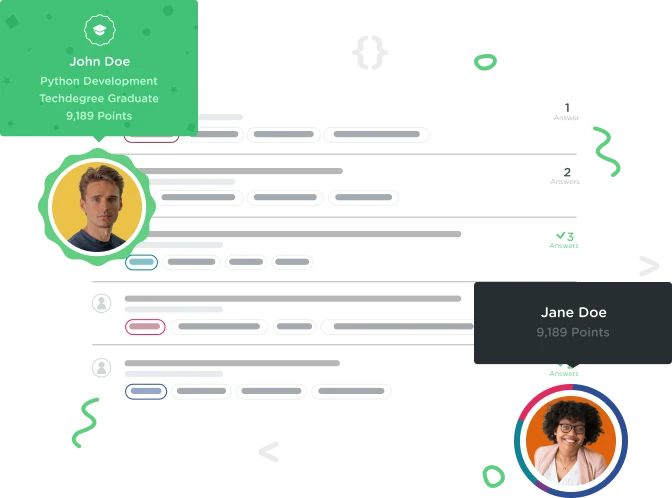

keith frazier
Python Development Techdegree Student 5,771 PointsNot sure if __int__ method or comparison methods are incorrect.
The Song challenge has been giving me trouble, and the code that ended up working has the same functionality as the code that I had been trying for hours. I cannot find where in my code the challenge saw a problem, when I run in other IDE's it works fine, including workspaces.
Here is my code:
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
def __int__(self):
if ":" in self.length:
minute = int(self.length[0])
second = int(self.length[2:4])
self.length = (minute * 60) + second
return (self.length)
def __eq__(self, other):
return self.__int__ == other
def __lt__(self, other):
return self.__int__ < other
def __gt__(self, other):
return self.__int__ > other
def __le__(self, other):
return self.__int__ <= other
def __ge__(self, other):
return self.__int__ >= other
And here is the code that the challenge is expecting:
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
def __int__(self):
return self.length
def __eg__(self, other):
return int(self) == other
def __lt__(self, other):
return int(self) < other
def __gt__(self, other):
return int(self) > other
def __le__(self, other):
return int(self) < other or int(self) == other
def __ge__(self, other):
return int(self) > other or int(self) == other
I see there are many differences but they do the exact same thing. I even added the option to convert a string into a time because there is no preview or any indication of what error is occurring.
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
1 Answer
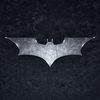
Logan R
22,989 PointsHi Keith,
The first thing that jumps out at me is that when you do your return self.__int__ == other
s, you need to add ()
to __int__
for each comparison.
If you don't, you will get a result like the following:
>>> s = Song("a", "b", 100)
>>> s.__int__
<bound method Song.__int__ of <__main__.Song object at 0x0000026CED7B7DA0>>
>>> s.__int__()
100
As for your int method, I strongly suspect that self.length is an integer. I suspect also for the challenge, they are manually inspecting your __int__
and making sure it is purely just self.length. I found that if I delete your ":"
if statement and add the ()
to each comparison, the challenge passes.