Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial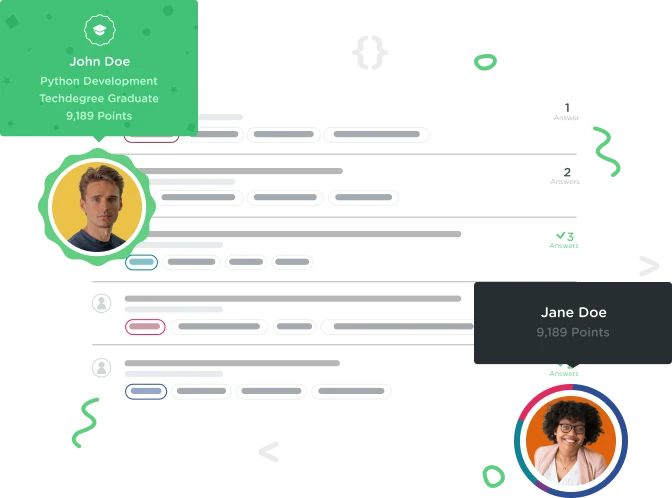

Kim Andersen
6,742 PointsNot sure what I am doing wrong, but the script won't take my price even though I input it as an int. Any ideas?
from models import(Base, session,
Book, engine)
import datetime
import csv
def menu():
while True:
print("""
\nAdd a Book
\rView All Books
\rSearch for a Book
\rBook Analysis
\rExit
""")
choice = input('What would you like to do? ')
if choice in ["1", "2", "3", "4", "5"]:
return choice
else:
input("""
\rPlease choose one of the options above
\rA number from 1-5
\rPress enter and try again""")
# import models
# main menu - add, search, analysis, exit, view
# add books to the database
# edit books
# delete books
# search books
# data cleaning
# loop runs program
def clean_date(date_str):
months = ['January', 'February', 'March', 'April', 'May', 'June',
'July', 'August', 'September', 'October', 'November', 'December']
split_date = date_str.split(" ")
try:
month = int(months.index(split_date[0]) +1)
day = int(split_date[1].split(",")[0])
year = int(split_date[2])
return_date = datetime.date(year, month, day)
except ValueError:
input("""
\n*** DATE ERROR ***
\rThe date format should include a valid Month Day, Year from the past
\rEX: January 13, 2003
\rPress enter to try again.
\r********************
""")
return
else:
return return_date
month = int(months.index(split_date[0]) +1)
day = int(split_date[1].split(",")[0])
year = int(split_date[2])
return
def clean_price(price_str):
try:
price_float = float(price_str)
except ValueError:
input("""
\n*** Price ERROR ***
\rThe price should be a number without a currency symbol
\rEX: 10.99
\rPress enter to try again.
\r********************
""")
else:
return int(price_float * 100)
def add_csv():
with open("suggested_books.csv") as csvfile:
data = csv.reader(csvfile)
for row in data:
book_in_db = session.query(Book).filter(Book.title==row[0]).one_or_none()
if book_in_db == None:
title = row[0]
author = row[1]
date = clean_date(row[2])
price = clean_price(row[3])
new_book = Book(title=title, author=author, published_date=date, price=price)
session.add(new_book)
session.commit()
def app():
app_running = True
while app_running:
choice = menu()
if choice == "1":
#add book
title = input("Title: ")
author = input("Author: ")
date_error = True
while date_error:
date = input("Published date (Ex: October 25, 2017): ")
date = clean_date(date)
if type(date) == datetime.date:
date_error = False
price_error = True
while price_error:
price = input("Price (Ex: 25.64): ")
price = clean_price(price)
if type(price) == int:
price_error = False
elif choice == "2":
#view book
pass
elif choice == "3":
#search book
pass
elif choice == "4":
# analysis
pass
else:
print("GOODBYE")
app_running = False
if __name__ == "__main__":
Base.metadata.create_all(engine)
app()
#add_csv()
#for book in session.query(Book):
# print(book)
1 Answer
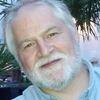
Jeff Muday
Treehouse Moderator 28,720 PointsYou have the beginning of a very nice app!
You forgot to put in the sentinel condition check, it would always loop because price_error always was False. I noticed you return a float
for correct input and None
for incorrect input. I simply check for a price that is "not None" and there you have it!
price_error = True
while price_error:
price = input("Price (Ex: 25.64): ")
price = clean_price(price)
# sentinel condition price is not None
if not (price is None):
price_error = False
Good luck with your Python journey!