Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial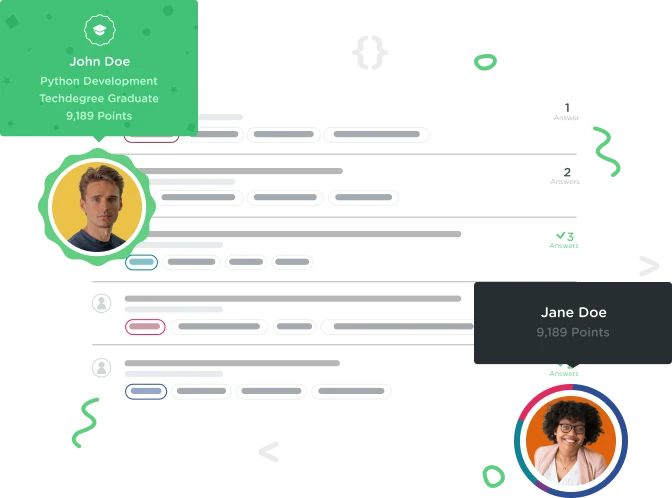

Richard Pitts
Courses Plus Student 1,243 PointsNot sure what I did wrong here!
Not sure what I did wrong here!
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int = 4
init(numberOfSeats: Int) {
self.numberOfSeats = numberOfSeats
}
}
let someCar = Car(numberOfSeats: 4)
1 Answer
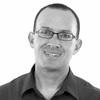
David Papandrew
8,386 PointsHi Richard,
Car is a subclass of Vehicle so you have to make sure that you initialize the superclass properties as well. You do this by using by calling super.init() in the subclass designated initializer.
Note that in the subclass designated initializer you must initialize all your subclass properties first and then you call the super.init.
Here is the code:
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
var numberOfSeats: Int = 4
init(withDoors doors: Int, andWheels wheels: Int, numberOfSeats: Int) {
self.numberOfSeats = numberOfSeats
super.init(withDoors: doors, andWheels: wheels)
}
}
// Enter your code below
let someCar = Car(withDoors: 4, andWheels: 4, numberOfSeats: 4)
Also worth noting that since you have a default value for the Car subclass' only stored property (numberOfSeats), you technically don't need an initializer for the subclass. You could get away with this solution where you simply use the inherited super class initializer when instantiating a new car object.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
var numberOfSeats: Int = 4
}
// Enter your code below
let someCar = Car(withDoors: 4, andWheels: 4)