Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial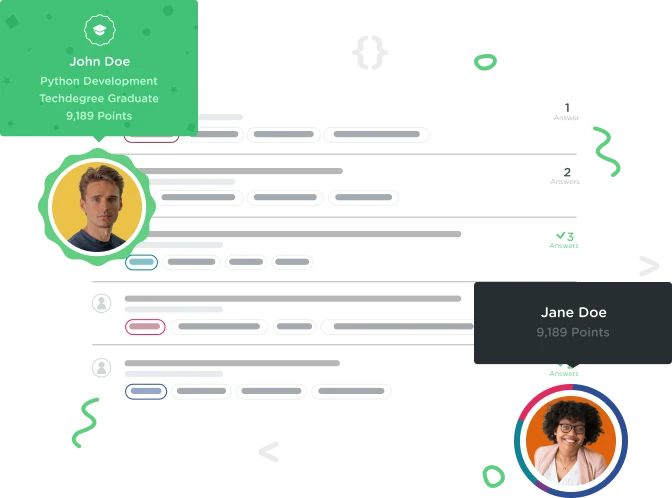
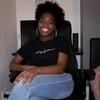
Kaleshe Alleyne-Vassel
8,466 PointsNot sure what I'm being asked to do. I've tried various methods and none of them seem to be working
It says to make the p element before the button have the class highlight when clicked. I can't see where I am going wrong and am unsure on how to go about it. I've tried a google search and what not, to no avail. Please help!
const list = document.getElementsByTagName('ul')[0];
const button = document.getElementsByTagName('button');
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
button.previousElementSibling.className = 'highlight';
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers

Heidi Puk Hermann
33,366 Pointsfirst of, you don't need to create the button-variable at line 2. it is not a part of the assignment (and will therefor create an error) but you don't need it.
Second the previousElementSibling
is a read-only property, so you cannot use it to alter the sibling <p>
-tag. Instead you should use the e.target
to tell what button is pushed and previousSibling
to get the <p>
-tag. Then your code will be:
...
e.target.previousSibling.className = "highlight";
...
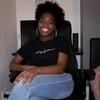
Kaleshe Alleyne-Vassel
8,466 PointsThanks a bunch, the explanation you added is very helpful too!

Heidi Puk Hermann
33,366 Points@Kaleshe, you're very welcome :)
@Mike - I've spent quite some time getting my head around the DOM and how to use it. I agree that it can be a lot of work, but on the other hand, once you get your head around it, you can really do some strong stuff rather "simple". And interesting that it does work with previousElementSibling
since the documentation said it is a read-only property... Well life is interesting :)
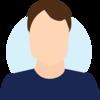
Mike Hatch
14,940 PointsHeidi, yes the DOM really is its own beast. It requires its own separate study: HTML/CSS/JS/DOM. I think Douglas Crockford lists it as an "awful" part of JS. I couldn't agree more.
Mike Hatch
14,940 PointsMike Hatch
14,940 PointsThis is a tough one. I tried to resolve it just now, but then saw you beat me to it. I struggle with the DOM in general. It is so much code for so little. It seems most students tried to solve it by adding multiple new lines of code when it is asking you to add just one. It does actually pass if you use
previousElementSibling
withevent.target
before it instead ofbutton
.