Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial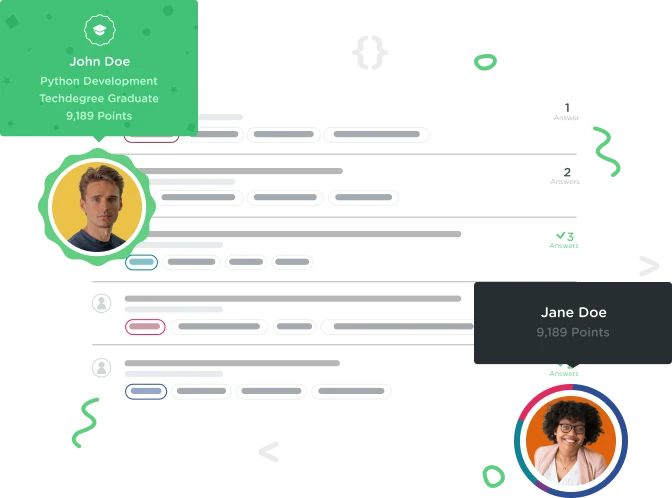

larsberg
58,676 PointsNot sure what I'm doing wrong
We want the First function to return the first element of the slice we pass in. But it's possible that First might receive an empty slice. So First should also return an error value. If slice is not empty, have First return the slice's first element, and an error value of nil. If slice is empty, have First return 0, and an error value whose string is set to "Slice is empty". You can use len(slice) to check whether the slice is empty, and fmt.Errorf("Slice is empty") will create the appropriate error value.
My Answer:
package utility
import "fmt"
func First(slice []int) (int, error) {
// YOUR CODE HERE
if len(slice) > 0 {
return slice[0], nil
}
else if len(slice) == 0 {
return 0, fmt.Errorf("Slice is empty")
}
}
1 Answer
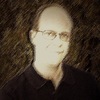
Jason Anders
Treehouse Moderator 145,860 PointsHey there,
You are really close. The only thing is you are using an else if
when you should be using only an else
. The if
is checking if the length is greater than 0... the only other option is zero or less, so if the fist conditional fails (i.e. the if
statement) then the else
clause will execute.
So, you are on the right track you just need to change the
else if len(slice) == 0 {
to just
else {
Also, for some reason (and I'm fairly new to Go
), but the challenge throws an error with your else statement on a new line. If you move it up to the same line as the closing curly bracket for the if
it works fine. ?? Tagging Jay McGavren for clarification if that return
really should cause an error?
So, what you need then is this:
package utility
import "fmt"
func First(slice []int) (int, error) {
if len(slice) > 0 {
return slice[0], nil
} else {
return 0, fmt.Errorf("Slice is empty")
}
}
Keep Coding! :)
Joe Purdy
23,237 PointsJoe Purdy
23,237 PointsThe short answer is that Go's compiler automatically injects semicolons and doesn't perform lookahead to allow for opening curly braces on separate lines. You can read more about this here: Why are there braces but no semicolons? And why can't I put the opening brace on the next line?
When you're writing Go it's recommended to use a tool like gofmt, goimports, or goreturns to format your code to the idiomatic style the Go compiler expects. Many IDEs have Go language plugins that can be installed and configured to run a utility like this automatically to always be formatting to the one true syntax.
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsThank you for the explanation and links Joe Purdy... Very helpful in understanding.
Jay McGavren
Treehouse TeacherJay McGavren
Treehouse TeacherWhat Joe Purdy said. :) I will have to explicitly mention the brace-on-same-line rule when we get to the Go Basics course.