Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial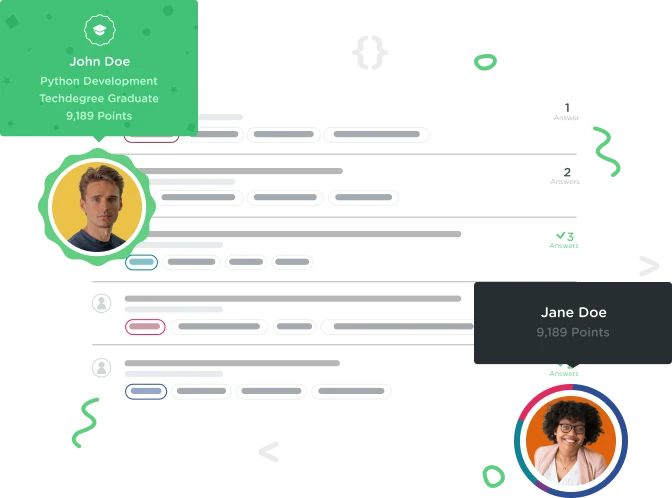
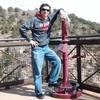
Aaron Hinesley
2,993 PointsNot sure what I'm doing wrong here.
Is there any easy way to test small chunks as I go along? Finding it hard to see what exactly I'm doing wrong.
def disemvowel(word):
word_list = word.split()
new_list = []
vowels = ['a', 'e', 'i', 'o', 'u']
for letter in word_list:
if letter.lower == vowels:
new_list.append(letter)
else:
continue
word = word.join(new_list)
return word
2 Answers

KRIS NIKOLAISEN
54,971 PointsYou can test small chunks by using print statements.
With your code you should run the following to see if it is what you intended:
def disemvowel(word):
word_list = word.split()
print(word_list)
new_list = []
vowels = ['a', 'e', 'i', 'o', 'u']
for letter in word_list:
print(letter)
if letter.lower == vowels:
new_list.append(letter)
else:
continue
print(new_list)
word = word.join(new_list)
return word
print(disemvowel('TrvEki'))
TrvEki was the word the checker used when entered into the challenge. You might be surprised to see in this case
word_list is ['TrvEki']
letter is TrvEki
new_list is []
Some hints:
- to create word_list use list(word)
- the lower method should be followed by parentheses
- you will want to append to new_list if letter is not in vowels
- join new_list with an empty string separator like this word = ''.join(new_list)

Blake Kazmierzak
2,491 PointsYou can download the latest version of Python for your operating system online and test your code in IDLE (integrated development and learning environment), the environment that's packaged with Python on the official website.
I think you're overcomplicating the challenge a bit. Try this:
def disemvowel(word):
return ''.join([letter for letter in word if letter.lower() not in ['a','e','i','o','u']])
This method reduces the solution to one line using a list comprehension. I believe treehouse has a course that covers this topic. You can break it down into chunks to understand what's going on. In English, this code returns a list of letters for any (forced lower-case) letter in word if that letter is not in the list of lower-case vowels. I then joined the list by an empty string and returned it, resulting in a single string with the expected results.