Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial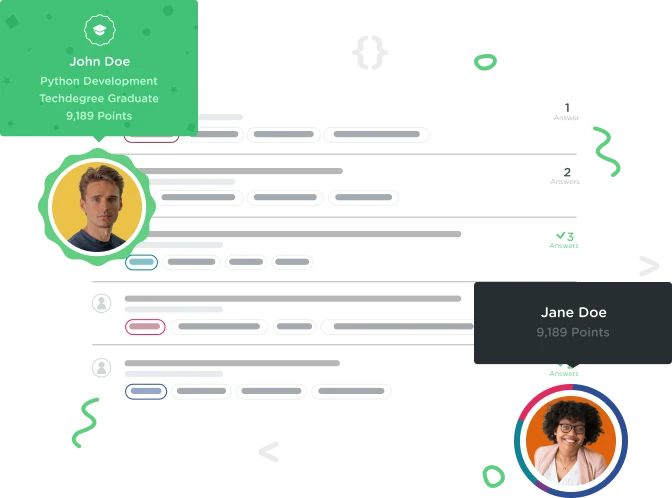
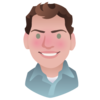
David Peyton
2,195 PointsNot sure what I'm doing wrong in this example
I guess it is obvious I don't need to type in the console.log to display words because we can't see the console when previewing.
So i'm not exactly sure about what i do next after setting the var up top
1 Answer
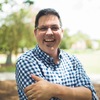
Dave McFarland
Treehouse TeacherIn this challenge you're supposed to use best practices for declaring variables inside a function. When setting up a "local variable" -- a variable that is only available to the function -- you should declare the variable with the var
keyword at the top of the function. However, you don't need to set its value there -- you should set the value of the variable wherever it makes sense in the function.
Here's an example of improperly declaring a local variable within a function:
function myFunction(val) {
if (val===true) {
var newVar = "This is a local variable";
}
}
See how the newVar
variable isn't declared until the conditional statement. Here's a better way to do it:
function myFunction(val) {
var newVar;
if (val===true) {
newVar = "This is a local variable";
}
}
The variable is declared at the top of the function, but it's value isn't set until inside the conditional statement. It isn't required that you declare local variables in this way, but it makes it a lot easier to identify local variables when your functions become more than just a few lines of code long.
Dave Evans
13,160 PointsDave Evans
13,160 PointsPlease post your script so that we can better analyze what you are attempting to use.