Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial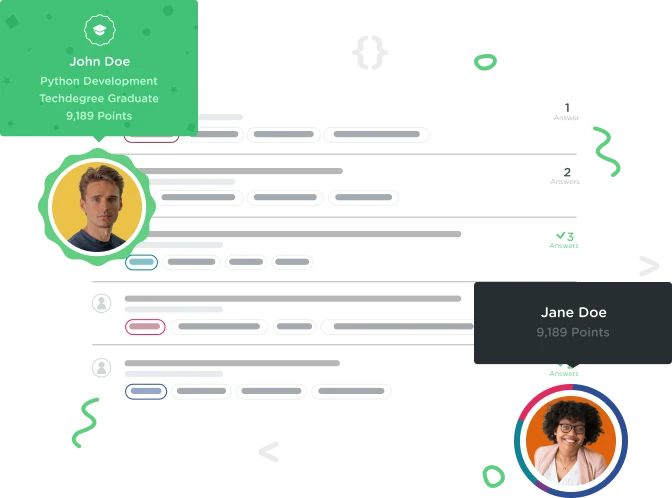

Adam Rosenbaum
5,258 PointsNot sure what I'm missing here. I get an error Ingredients.get() does not return a string. I've even tried return str()
It has got to be something small I just can't see it.
import datetime
from peewee import *
DATABASE = SqliteDatabase('recipes.db')
class Recipe(Model):
name = CharField()
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
class Ingredient(Model):
name = CharField()
description = CharField()
quantity = DecimalField()
measurement_type = CharField()
recipe = ForeignKeyField(Recipe)
class Meta:
database = DATABASE
# TODO: Ingredient model
# name - string (e.g. "carrots")
# description - string (e.g. "chopped")
# quantity - decimal (e.g. ".25")
# measurement_type - string (e.g. "cups")
# recipe - foreign key
from flask.ext.restful import Resource
import models
class IngredientList(Resource):
def get(self, id):
return 'anything you want'
class Ingredient(Resource):
def get(self, id):
return 'anything you want'
2 Answers

Myers Carpenter
6,421 PointsI think you are missing that it needs to be a class method. Here's an example of one:
class IngredientList(Resource):
# this is an instance method. You can only call it if you have a instance of IngredientList. (e.g.: IngredientList().get(1))
def get(self, id):
return 'anything you want'
# This is a class method. (e.g. IngredientList.get(1))
@classmethod
def get(self, id):
return 'anything you want'
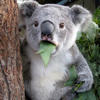
ursaminor
11,271 PointsIt doesn't have to be a class method. I copied your code into the challenge and the error message is "IngredientList.get()` should return a string", not "Ingredients.get() does not return a string". The problem is IngredientList.get() does not take id. Take out id and it passes.