Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial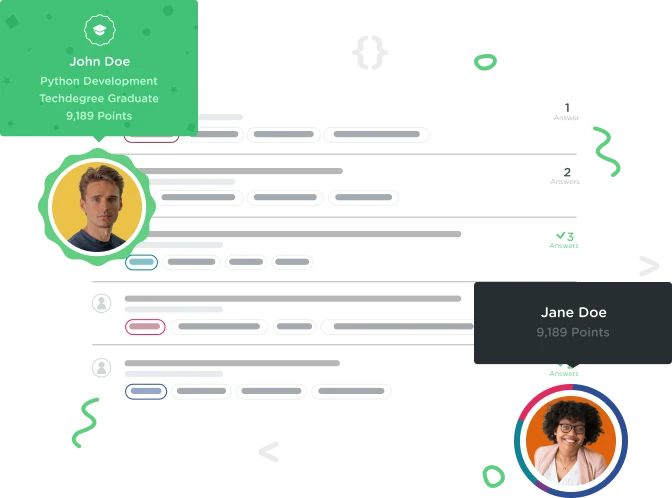

robert morea
7,599 PointsNot sure what is wrong here ...
So...I'm sure it's something simple but I'm not sure what is wrong here. The 'error hint' even says 'Bummer! You need to call next()
on line 11.' ... which I'm pretty sure I'm doing. It starts with a $, ends with a semicolon, includes the (), and all the other stupid errors I can think of.
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
//Hide hints
$("form span").hide();
function passwordEvent(){
$("#password").focus(function(){
if($(this).val().length > 8) {
//Hide hint if valid
$(this).next().hide();
} else {
//else show hint
$(this).next().show();
}
});
}
//When event happens on password input
$("#password").focus(passwordEvent).keyup(passwordEvent);
//When event happens on confirmation input
//Find out if password and confirmation match
//Hide hint if match
//else show hint
<!DOCTYPE html>
<html>
<head>
<title>Sign Up Form</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<form action="#" method="post">
<p>
<label for="username">Username</label>
<input id="username" name="username" type="text">
</p>
<p>
<label for="password">Password</label>
<input id="password" name="password" type="password">
<span>Enter a password longer than 8 characters</span>
</p>
<p>
<label for="confirm_password">Confirm Password</label>
<input id="confirm_password" name="confirm_password" type="password">
<span>Please confirm your password</span>
</p>
<p>
<input type="submit" value="SUBMIT">
</p>
</form>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
robert morea
7,599 Pointsrobert morea
7,599 PointsActually, I thought I had to put in the .focus statement on line 8, but it isn't needed. Take it out and everything works.
I do think the 'hints' in this section have been a bit off. I quite often get 'task 1 is no longer passing,' which is uninformative (or even, near as I can tell, not correct, in that task 1 should be unaffected), and now this one is also not quite right, because the problem wasn't on line 11 and didn't involve a missing call to .next().
But anyway, it works now.