Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial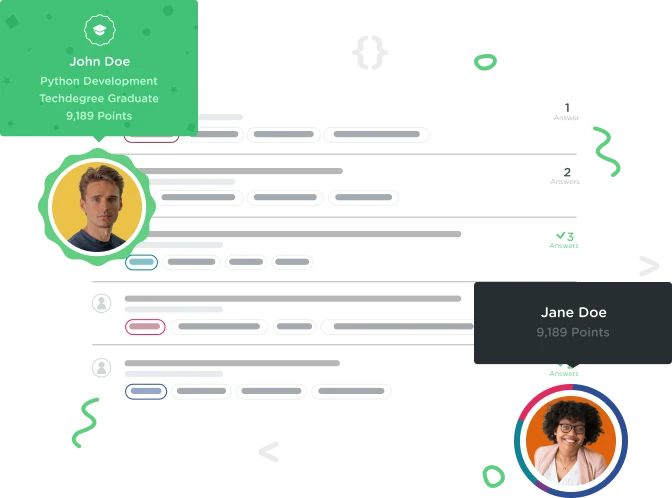
Ryan Doyle
8,587 PointsNot sure what is wrong with my for the challenge. Struggling with the "self" portions of the code, I think...
Ok, there is a challenge in the Object-Oriented 2.0 that asks you to do this...
*In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness.*
Here is what I did:
struct Location {
let latitude: Double
let longitude: Double
init(latitude: Double, longitude: Double) {
self.latitude = latitude
self.longitude = longitude
}
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = Location(latitude: <#T##Double#>, longitude: <#T##Double#>)
}
}
let someBusiness = Business(name: "REI", Location(latitude: 12.1, longitude: 12.2)
I felt pretty confident typing it but now that it's wrong I have no clue what to do. I am thinking I might be misunderstanding the "self" stuff. As I was writing it, I was having a hard time trying to figure out how to initialize an instance of the location by entering in the lat and long, so maybe that's what's wrong....any help would be awesome! These lessons in swift have been a challenge. Also first time posting so, sorry if the markdown makes everything look a little off...
2 Answers
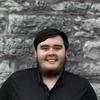
Michael Hulet
47,912 PointsThere's only 2 things I see wrong with your code. Overall, I think you understand the self
keyword properly. The 2 mistakes I see are that in the initializer for Business
, it's gonna be way easier to just assign self.location = location
instead of initializing a whole new Location
instance. The other one is a really simple mistake that I make all the time, even after 2 years of writing Swift. You just forgot a closing paren at the very end of the last line, so it should read like this:
let someBusiness = Business(name: "REI", Location(latitude: 12.1, longitude: 12.2))

Mohammed Abushamala
3,650 Pointsstruct Location { let latitude: Double let longitude: Double }
class Business { let name: String let location: Location
init(name: String, location: Location){
self.name = name
self.location = Location(latitude: 20.9, longitude: 29.9)
}
}
let someBusiness = Business(name: "REI", location: Location(latitude: 12.1, longitude: 12.2))
Ryan Doyle
8,587 PointsRyan Doyle
8,587 PointsYes! It worked! Thank you. I should have noticed that last ) but I think I was caught up on having the Location parts set up incorrectly.
I don't think I was putting together that by initializing an instance of Business, the self.location = location would go to the location of the Business class, which I had set up as a "Location" type. I was thinking at some point in initializing I would have to enter in the lat/long, but I suppose that's the point of making the struct in the first place.
Thank you!