Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial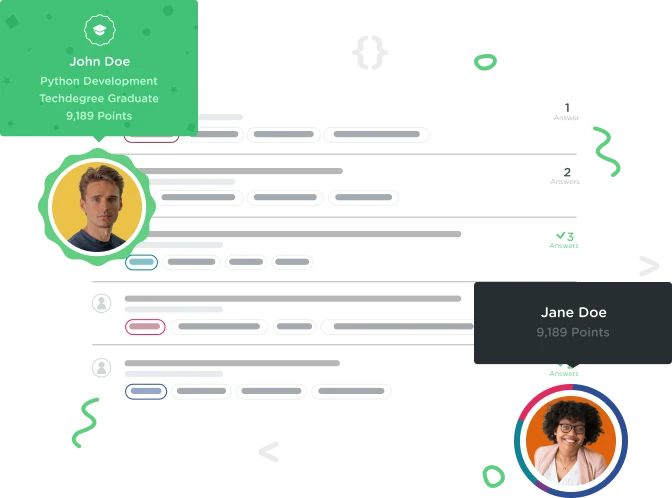

Susan Rusie
10,671 PointsNot sure what is wrong with this code for Fixing the DOM Manipulation Code Challenge. Please Help!
What am I doing wrong? It says "Syntax error: app.js: Unexpected token 'l' (10:11)" Here is my code:
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
if law.className === 'embolden') {
law.style.display = '';
// replace 'false' with a correct test condition on the line below
if (false) {
for (let i = 0; laws.length; i =+ 1;) {
let li = laws[i];
laws.style.display = '';
}
}
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});

Susan Rusie
10,671 PointsI made those changes as suggested, but it still didn't work. Then, I tried this too and it said it had a reference error: can't find tagName. I don't understand what I am doing wrong.
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
if (tagName === 'li') {
let law = laws[i];
law.style.fontWeight = 'bold';
}
}
if (false) {
for (let i = 0; laws.length; i += 1) {
let law = laws[i];
law.style.fontWeight = 'normal';
}
}
});
2 Answers

Susan Rusie
10,671 PointsI finally figured it out. I quit thinking so hard about it and it finally came to me. All I had to do was change the following:
if (false) {
to
if (index === i) {
Once I made that change, I passed the challenge.
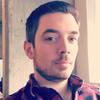
Garett Haight
16,632 PointsThat's right, tagName isn't a defined variable. If you wanted to check the tag name here, you would use: laws[i].tagName, but it looks like this is unnecessary since getElementsByTagName() should only return li elements. Also, I'm not sure what the if statement below that is supposed to be doing, but the code in that block will never run since false will never be true.
Garett Haight
16,632 PointsGarett Haight
16,632 PointsYou forgot your opening parenthesis on line 10:
if law.className === 'embolden') {
fixed: if (law.className === 'embolden') {
Also, on line 14: for (let i = 0; laws.length; i =+ 1;)
the last semicolon is causing a syntax error: for (let i = 0; laws.length; i =+ 1) {
The error actually gives you the location of the syntax error (10:11) line 10, col 11