Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial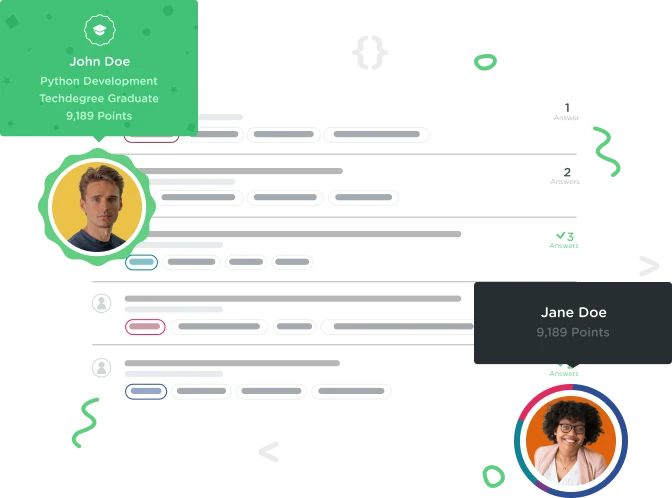

tyler borg
9,429 PointsNot sure what to do
I really didn't even know where to start on this challenge...I feel like I am not actually understanding any of this OOP.
Challenge Task 1 of 1 Alright, here's a fun task!
Create a function named combiner that takes a single argument, which will be a list made up of strings and numbers.
Return a single string that is a combination of all of the strings in the list and then the sum of all of the numbers. For example, with the input ["apple", 5.2, "dog", 8], combiner would return "appledog13.2". Be sure to use isinstance to solve this as I might try to trick you.
def combiner(args):
words = []
nums = []
for item in args:
if isinstance(item, str):
words += item)
if isinstance(item, (int, float)):
nums += item
return words + str(nums)
2 Answers
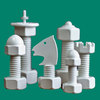
Steven Parker
241,809 PointsYou're close, but based on how they are being used in the rest of the code, neither of the accumulators should start off as an empty list. One is a string, the other is a number:
words = ""
nums = 0
Also, there's a stray closing parenthesis at the end of the "words += item
" line.

tyler borg
9,429 PointsThanks guys
Jimmy Sweeney
5,649 PointsJimmy Sweeney
5,649 PointsI ended up solving this challenge by starting off the accumulators as empty lists.
Tyler, if you are going to start off words and nums as empty lists, then you'll need to add the items to those lists as you cycle through the for-loop.
In pseudocode:
If the item from the original list is a string, append it to words.
If the item from the original list is an int or float, append it to nums.
Sum the numbers and join the strings
Return: Concatenation off those things from the line above.
Of course, it would be a little simpler if you end up going with Steven's route :)