Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial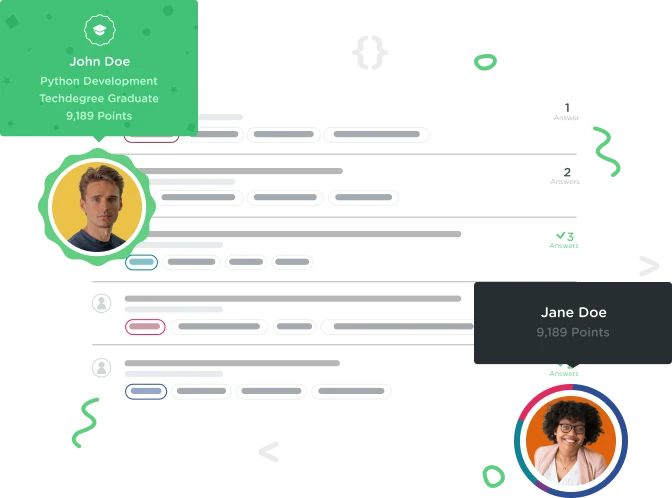
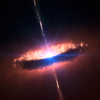
Brad Lait
8,343 PointsNot sure what to do
It asks you to fix it so that 'x' is still 1 in the global scope. pleas help
var x = 1; var y = 1;
function hypotenuse(a , b) {
var cSquared = a * a + b * b;
x = Math.sqrt(cSquared);
return x;
}
hypotenuse(x, y);
5 Answers

Gareth Borcherds
9,372 PointsBecause the var x = 1; is set outside of a function, it because a global variable and because you use x without stating var x = Math.sqrt(cSquared); then JavaScript assumes you are using the global variable.
To complete the task you can either add var in front of the x inside the function, like so:
function hypotenuse(a , b) {
var cSquared = a * a + b * b;
var x = Math.sqrt(cSquared);
return x;
}
hypotenuse(x, y);
Or you can simply define a completely different variable like so
function hypotenuse(a , b) {
var cSquared = a * a + b * b;
var z = Math.sqrt(cSquared);
return z;
}
hypotenuse(x, y);
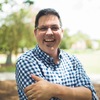
Dave McFarland
Treehouse TeacherTo create a variable that's local to a function, you need to add the var
keyword inside the function. In this example, there's a variable named x
outside the function -- in the "global" space -- and that same variable is used inside the function. This changes the value of x
in the global space. In other words, this code
x = Math.sqrt(cSquared);
Changes the value of x
outside the function, but this code
var x = Math.sqrt(cSquared);
Creates a new local variable named x
which doesn't affect the outer, global x
variable.
Hope that helps. If not, try watching this video again: http://teamtreehouse.com/library/javascript-foundations/variables/scope-2
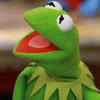
Tommy Gebru
30,164 Pointsisnt the difference between global and local mean the placement, whether it is inside or outside the function of block code?
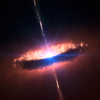
Brad Lait
8,343 Pointsyes
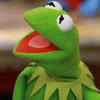
Tommy Gebru
30,164 Pointsso in this case would you call x outside of the function just above the hypotenuse or below it?
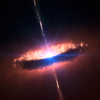
Brad Lait
8,343 Pointsvar x = 1; var y = 1;
function hypotenuse(a , b) {
var cSquared = a * a + b * b;
x = Math.sqrt(cSquared);
return x;
}
var x = 1;
hypotenuse(x, y);
but it says "The global variable x was 1.4142135623730951 not 1"

Jason Saba
Courses Plus Student 3,862 PointsYou're almost there, below it. It's in the right place as far as whether or not it needed to be inside/outside the function, but since the it declared ABOVE the hypotenuse function call, when the hypotenuse function runs it changes the value, so you just need to move the global variable below the function call in order to not affect it's value. Though that's not exactly the correct way.