Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial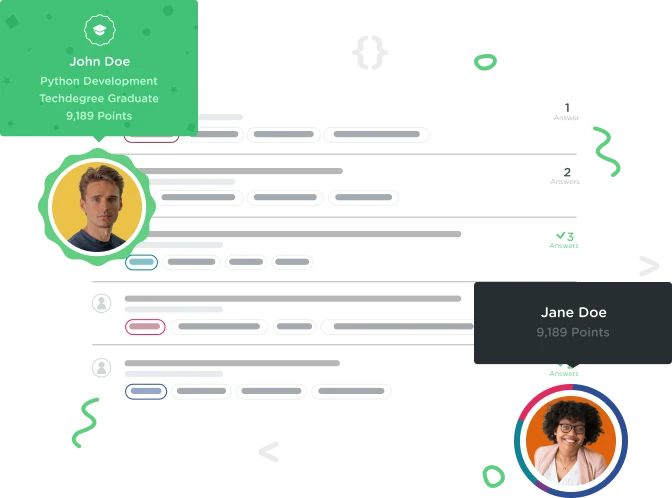
Thomas Morling
12,754 PointsNot sure what to do for this challenge
Unclear what to do
<?php
include "helper.php";
/*
* helper contains the following variables:
* $item is an array that contains details about the library item
* $results is a PDOstatement object with our genre results.
*/
1 Answer

Colin V
5,784 Points$item is an array that contains details about the media item.
$results is a PDOstatement object with our genre results.
Fetch the associative array of $results to loop through, and add an additional "genres" array element to $item. This "genres" element should be an internal associative array with the key of genre_id and the value of genre.
Example $item array:
<?php array(8) { ... ["genres"]=> array(2) { [3]=> string(6) "Comedy" [16]=> string(6) "Sci-Fi" } }
Lets break this down. It tells us that $item is an array that contains details about the media item. To make it more clear, you can test this out yourself and do var_dump($item) and preview, you should see something like this on the screen:
array(7) { ["title"]=> string(50) "Refactoring: Improving the Design of Existing Code" ["category"]=> string(5) "Books" ["img"]=> string(25) "img/media/refactoring.jpg" ["format"]=> string(9) "Hardcover" ["year"]=> string(4) "1999" ["publisher"]=> string(27) "Addison-Wesley Professional" ["isbn"]=> string(14) "978-0201485677" } Alright, so that there is definitely an array about a book, and it tells us exactly what's in the array.
Then it says $results is a PDOstatement object with genre results. What's that mean? Again, check with var_dump:
object(PDOStatement)#3 (1) { ["queryString"]=> string(217) "SELECT * FROM Media JOIN Media_Genres ON Media.media_id = Media_Genres.media_id JOIN Genres ON Media_Genres.genre_id = Genres.genre_id WHERE Media.media_id=?" } and there's our sql query.
From there, it says to loop through the results object. We learned from the previous videos to do that we would use a while loop, like so:
<?php
while($row = $results->fetch(PDO::FETCH_ASSOC)) {
}
Alright cool, but what data is being processed? Again, var_dump coming in in the clutch:
array(8) { ["media_id"]=> string(1) "3" ["title"]=> string(50) "Refactoring: Improving the Design of Existing Code" ["img"]=> string(25) "img/media/refactoring.jpg" ["genre_id"]=> string(2) "17" ["format"]=> string(9) "Hardcover" ["year"]=> string(4) "1999" ["category"]=> string(5) "Books" ["genre"]=> string(4) "Tech" } array(8) { ["media_id"]=> string(1) "3" ["title"]=> string(50) "Refactoring: Improving the Design of Existing Code" ["img"]=> string(25) "img/media/refactoring.jpg" ["genre_id"]=> string(2) "51" ["format"]=> string(9) "Hardcover" ["year"]=> string(4) "1999" ["category"]=> string(5) "Books" ["genre"]=> string(8) "Business" }
Alright! here's the array we need to add the genre id key and genre value pair to the items array. This is something also learned in the video, but simply put:
<?php
$item[$key] = $value
And for the genre array key to have an associative array as a it's value, you just make the value an array!
<?php
$assocarray[$key] = $value;
So if you put it all together, we should get something like:
<?php
while($row = $results->fetch(PDO::FETCH_ASSOC)) {
$genreID = $row["genre_id"];
$genre = $row["genre"];
$genreArr[$genreID] = $genre;
$item["genres"] = $genreArr;
}
return $item;
If you're confused about any of this, rewatch the prior video's, they help a lot! (this isn't the only way to do this, so be open to doing it in other better/worse ways!)
tommyc
1,966 Pointstommyc
1,966 PointsColin V A very helpful and easy to follow walkthrough. Thank you for taking the time. :)