Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial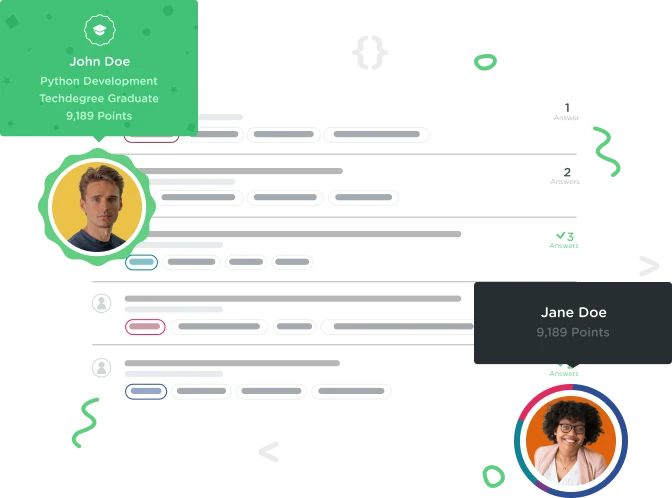

Michael LaCroix
5,828 PointsNot sure what "User" is
If you run the code and look at the error message it says it's looking for a User, String, String. I don't remember ever learning what a User is. I googled it and found nothing so I'm not sure what I'm supposed to be giving it. All I can see is that it's returning mAuthor but that doesn't seem to be returning anything. I tried making mAuthor = author several lines below that, but it didn't seem to work. Thoughts?
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public ForumPost(User author, String title, String description){
mTitle = title;
mDescription = description;
}
public String getDescription(){
return mDescription;
}
// TODO: We need to expose the description
}
public class User {
private String firstName;
private String lastName;
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
// TODO: Set the private fields here
}
public String getFirstName(){
return mFirstName;
}
public String getLastName(){
return mLastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum (String topic){
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Coding");
// Take the first two elements passed args
User author = new User("Michael", "LaCroix");
// Add the author, title and description
ForumPost post = new ForumPost( "Getting Somewhere", "Journey with me as I learn coding.");
forum.addPost(post);
}
}
2 Answers
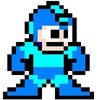
Robert Richey
Courses Plus Student 16,352 PointsHi Michael LaCroix,
User
is a type, just like String
is a type. User
is defined in User.java
and is used to create new objects which are instances of the User
class.
In Example.java
, you're creating a new User
object called author
by passing in your first and last name. Then, getting to the error, the ForumPost
constructor is expecting 3 arguments in this order User, String, String
. To fix this particular error, pass in author
as the first argument.
There will be more errors. If you find yourself hitting another wall, don't hesitate to ask. Be sure to pay attention to the comments in Example.java
as well as the error messages, as they are quite helpful.
Cheers

Michael LaCroix
5,828 PointsThanks. Didn't realize I still had to do some more work in ForumPost.java.
I'm not sure if this site isn't that great at giving directions or if I'm just super green and still learning to just read the directions properly in the first place. Can't tell you how many times in that course I was doing the wrong thing because I misunderstood the directions. Blaahh...
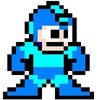
Robert Richey
Courses Plus Student 16,352 PointsI've found that when I take my time to read the question and understand what is being asked of me, the challenge questions and answers sync up. There have been a couple caveats to this, but by and large these challenges have been a very positive learning experience.
Especially this last Java challenge, because we have to essentially debug our way to the answer - which is what real world application development is like. You have some goal or expected outcome, and along the way there will be errors and you'll need to know how to debug them.
Think of this as the beginning of removing the training wheels.