Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial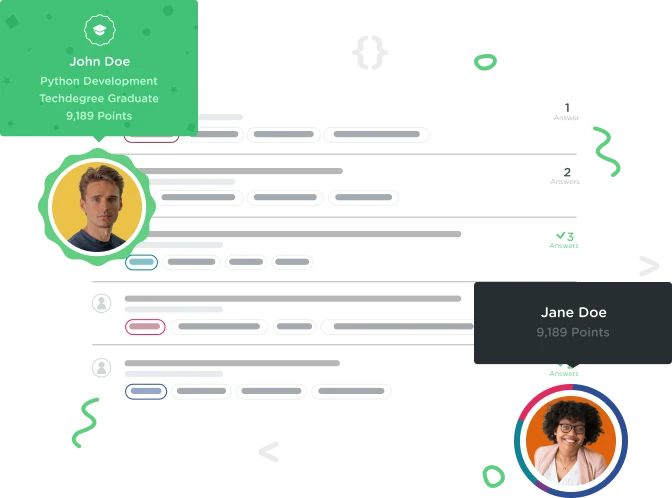

Agustin Fitipaldi
1,644 Pointsnot sure whats wrong here...
tried to do this squared function, but ended up confused.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
num = "2"
def squared(num):
try:
int(num)
except ValueError:
return num * len(num)
else:
return num * num
3 Answers
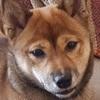
Katie Wood
19,141 PointsHi there,
Your code is mostly correct, and will work in most situations. The issue occurs when you feed in a number as a string, like your example ("2"). Let's walk through why. Say we call squared using "2" - we'd get here first:
try:
int(num) #this will pass, so it will skip the ValueError and go to the else block
else:
return num * num #here's where the issue happens
This will cause an issue because, while it did determine that num could be converted to an integer, the actual value of num is still "2". That means it is still trying to multiply the number as a string.
The fix that is closest to what you've got now would be to change the int(num) line into:
num = int(num) #would set the value also, so it will work when it tries to multiply
You have some other options, though. Here's one:
def squared(num):
try:
return int(num) * int(num)
except ValueError:
return num * len(num)
Hope this helps!
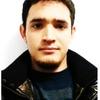
Anibal Marquina
9,523 Points# Check you Try block.
# If the argument (arg) can be turned into an integer then is a number... return the square value of that argument.
# If the argument (arg) cannot be turned into an integer.. then return the argument multiplied by its length.
def squared(arg):
try:
int(arg)
return int(arg) ** 2
except:
return arg* len(arg)
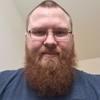
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHere is another solution if you prefer to use the else block
def squared(num):
try:
num = int(num) # add 'num =' here :-)
except ValueError:
return num * len(num)
else:
return num * num