Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial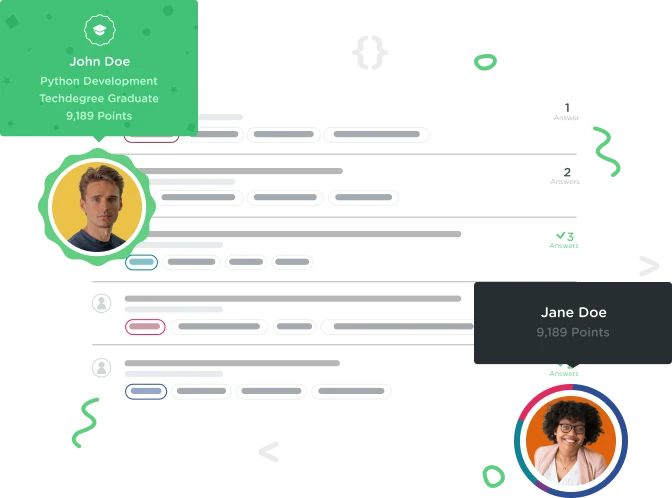

michael edmondson
4,511 PointsNot sure where im stuck at here
Can somoone please assist me with the error im making here ?
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
if(e.target.className == 'highlight'){
let li = e.target.parentNode;
let prevLi = li.previousElementSibling;
let list = li.parentNode;
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p class = "hightlight">Element Selection</p><button>Highlight</button></li>
<li><p class = "highlight">Events</p><button>Highlight</button></li>
<li><p class = "highlight">Event Listening</p><button>Highlight</button></li>
<li><p class = "highLight">DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
1 Answer
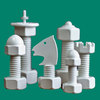
Steven Parker
231,248 PointsHere's a few hints:
- you don't need to test the current class setting
- you won't need to reference the parent for anything
- the paragraph is the sibling of the button itself
- once you traverse to the sibling, you'll set the class on it
- you can traverse and set the class in a single statement
michael edmondson
4,511 Pointsmichael edmondson
4,511 Pointssorry im still not getting you here still stuck
Steven Parker
231,248 PointsSteven Parker
231,248 PointsDo any of the hints make sense? Try to incorporate as many as you can, and then show the new state of the code and we can continue from there.
michael edmondson
4,511 Pointsmichael edmondson
4,511 Pointsvar list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { if (e.target.className == 'highlight'){ let prevLi = li.previousElementSibling; }
} });
Steven Parker
231,248 PointsSteven Parker
231,248 PointsLet me see if I can explain the hints better, and also include them into the code:
The final hint "you can traverse and set the class in a single statement" just means that you can combine the code that gets the sibling with the code that sets the class into one line. But this isn't necessary to pass the challenge.
michael edmondson
4,511 Pointsmichael edmondson
4,511 PointsStill Stuck this is my code. what am i missing here? i even rewatch the videos i dont think i get what being asked here var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { let p = event.target.parentNode; let pPrev = p.previousElementSibling; p.className = "highlight";
} });
Steven Parker
231,248 PointsSteven Parker
231,248 PointsRemember the 2nd hint, "you won't need to reference the parent for anything". The paragraph is the sibling of the button..
And you can't have two assignment operators in the same statement (but you don't need to create a variable at all if you assign the element directly).