Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial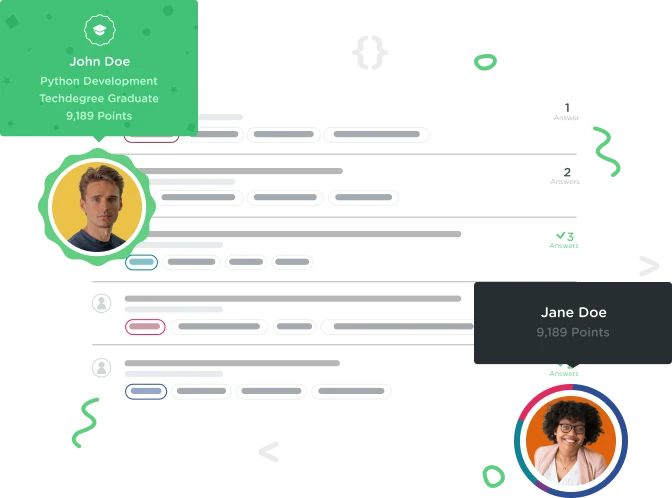
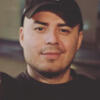
victor E
19,146 Pointsnot sure where to go from here
what am I missing on this reduce function?
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
hobbies = customers
.map(i => i.hobbies)
.reduce((arr, items) => [ ...arr, ...items ], []);
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
1 Answer
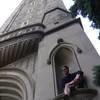
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHey Victor. You are not the only person having trouble with this challenge. I actually just answered another question about the same one, and both you and this other student made the mistake of thinking you need to use map
, which you don't. The Array Iteration Methods course does emphasize a lot that you can combine array iteration methods to perform complex data manipulations and I think students start to get it in their heads that they need to always be combining these methods. But I can tell you as someone who has built a bunch of apps over the past couple years, I don't actually use combinations of array methods as often as you would think. It's good to know how because in interviews you might be asked to solve tricky code challenges and require elegant and efficient solutions, but practical programming is often not as hard as the programming you're asked to do in interviews.
First, get rid of the map
call. reduce
does not need you to map over your data to simplify it first. reduce
can easily deal with complex data structures. Second, use the spread operator to add each item in the personal.hobbies
array to the accumulator. So your code will look like this:
hobbies = customers.reduce((arr, items) => [ ...arr, ...items.personal.hobbies ], []);
Since items
is your accumulator, I would rename it to something like curr
or currentItem
to help you remember the role of each parameter in reduce
, but you don't have to if you don't think it will help you.
As you can see, your code was really similar to mine, so you were definitely on the right track. Good luck!
victor E
19,146 Pointsvictor E
19,146 PointsThank you so much for taking the time to provide me with such a full answer. It is very helpful and will help me not overthink it in the future.Thank you!