Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial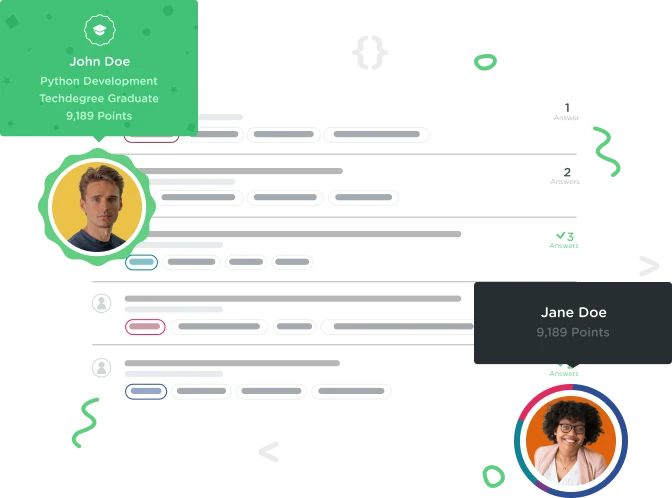

Ryan Munsch
6,552 PointsNot sure where to go with my code.
I have no clue how to structure the code for this challenge. Just asking for someone to point out where I'm going wrong and point me in the right direction. Thanks!
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
def random_item("Munsch"):
ind= random.randint(random_item)
return ind
1 Answer
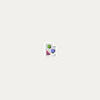
doesitmatter
12,885 PointsHi Ryan Munsch,
Lets start with importing the library:
import random
Then we define the function, we are told that it takes an iterable as an argument, lets call this itr
. In your code you passed an iterable as well "Munsch"
, only this isn't a variable iterable, a function always needs to have a variable argument, this way it is more useful to use, besides it is illegal in python for it not to be variable. So then our code would look like this:
import random
def random_item(itr):
Now we should figure out how we can get a random index from this iterable, the item which is at this random index will then be returned by our function. For this we use the random.randint
function, built into the random
library which we imported. This function takes two arguments, the first is the lowerbound, which will be included in the list of possible numbers which can be returned and the second is the upperbound which will also be included in the list of possible numbers which can be returned. For us the lowerbound is 0 and the upperbound is the length of the list - 1 (because we started counting at 0, there is no element at the index of the length of the list). This can be written like so:
import random
def random_item(itr):
ind = random.randint(0, len(itr) - 1)
Now all we need to do is return the element within the iterable itr
with the index of ind
:
import random
def random_item(itr):
ind = random.randint(0, len(itr) - 1)
return itr[ind]
That's it. Check out the documentation for more info on the randint()
function.
I hope this helped!