Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial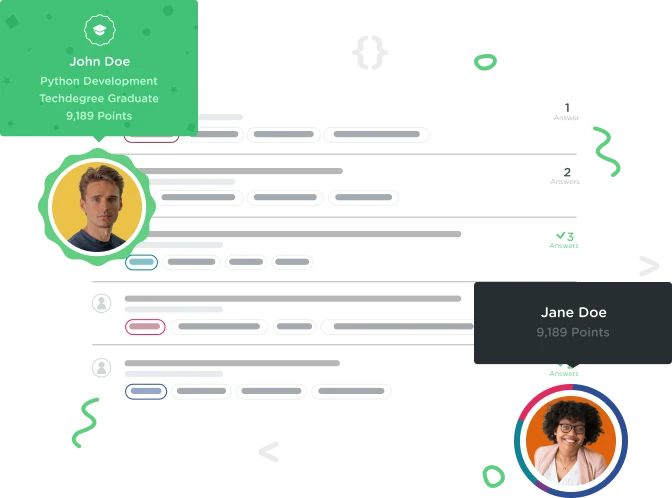

Peter Kurkowski
595 PointsNot sure where to start with this while loop question.
The question is:
"Okay, so let's use our new isFullyCharged helper method to change our implementation details of the charge method. Let's make it so it will only charge until the battery reports being fully charged. Let's use the ! symbol and a while loop. Inside the loop increment mBarsCount."
I honestly dont even know where to begin with this. Any help is appreciated.
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
1 Answer

Kaitlyn Brown
1,072 PointsI don't "speak" Java specifically - not for a long time now, anyway - but I can help you through the logic.
As you learn to program, you will start to find it easier to "translate" everyday words into programming notation. In this case, "Let's make it so it will only charge until the battery reports being fully charged" translates to, "Charge while the battery is not fully charged." So you're going to use the while
keyword with the !isFullyCharged
condition (because you only want things to happen when it's not fully charged, which is why you use the !
). Then, inside the loop, just write a single line to increment mBarsCount
, which probably looks something like mBarsCount++
See if you can take that logic and put it into Java syntax! I'm sure some other nice commenter here is more familiar with the syntax than I am if you get stuck.

Peter Kurkowski
595 PointsYou did a great job at explaining this. This worked for me. Being new to this is proving to be difficult! Almost every challenge i need to get help.
public void charge() { while (!isFullyCharged()) { mBarsCount++; } }
Mark Miller
45,831 PointsMark Miller
45,831 PointsThe charge() method needs a while loop, where you will use the not operator ! and the helper method isFullyCharged() to make the test condition for the while loop. Increment mBarsCount while it is not fully charged. The newly modified method now performs a real reloading, instead of a forceful assignment.