Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial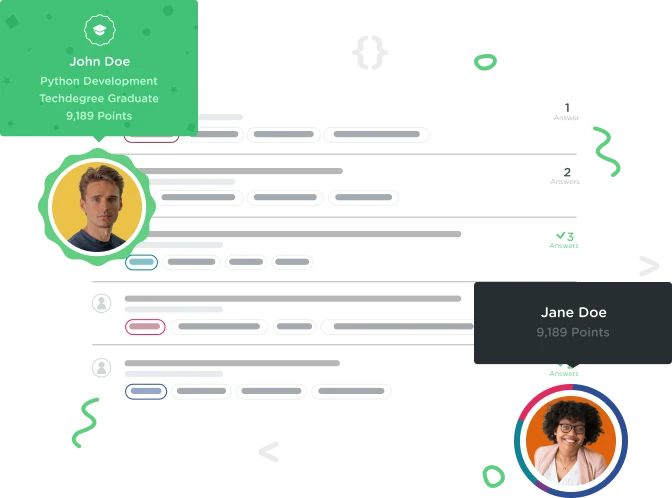

William Lee
1,316 PointsNot sure which part of my code is incorrect.
Yep.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n {
case n%3 == 0 && n%5 != 0: return "Fizz"
case n%5 == 0 && n%3 != 0: return "Buzz"
case n%5 == 0 && n%3 == 0: return "FizzBuzz"
}
// End code
return "\(n)"
}
1 Answer
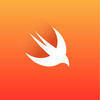
Steven Deutsch
21,046 PointsHey William Lee,
The problem with your solution is that your case statements are evaluating to a Boolean and you are trying to match that Bool to an Int value. If you want to use a switch statement to solve FizzBuzz we have to make a few tweaks to your code. There's a bunch of creative ways we can do this but one would be to use a "where" clause to check for additional conditions in the switch.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
/* Now your switch statement is checking for values of n where they meet
the specified conditions. */
switch n {
case n where (n%5 == 0 ) && (n%3 == 0): return "FizzBuzz"
case n where (n%3 == 0): return "Fizz"
case n where (n%5 == 0): return "Buzz"
default: break
}
// End code
return "\(n)"
}
Next, I also added a default case to your switch because switch statements must be exhaustive. I used the break statement to be implicit in stating that we don't want to do any functionality if the default case is matched.
Finally, I refactored your code to check for FizzBuzz first. This removes the need to check if numbers that are multiples of 3 are also multiples of 5 and vice versa, because if they were the switch would have already matched the first case and exited the statement.
Good Luck!