Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial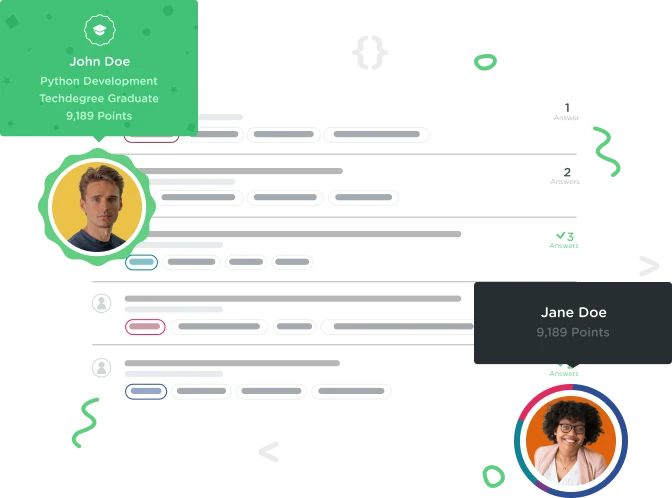
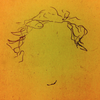
bristel
1,252 PointsNot sure why code doesn't work.
function max(x,y) { if (x > y) return x; } else { return y; };
max(4,6);
function max(x,y) {
if (x > y)
return x;
} else {
return y;
};
max(4,6);
5 Answers

Nicholas Hebb
18,872 PointsThe curly braces should be more like this:
function max(x,y) {
if (x > y) {
return x;
} else {
return y;
}
}
You can also shorten this using a ternary operator [1]:
function max(x, y) {
return x > y ? x : y;
}
[1] https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator

Nicholas Hebb
18,872 PointsHint: Look at your curly braces.
Also, the semicolon after the function declaration is unneeded.
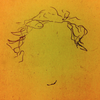
bristel
1,252 PointsHi Nicholas, thanks for the quick reply. I like the hints better than just the answer! I made the curly brace change and removed semi colon after the function declaration. Still having some trouble. This is what i have now.
function max(x,y) if (x > y) { return x; } else { return y; };
max(4,6)
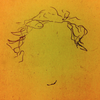
bristel
1,252 Pointsits in the correct format though. :)
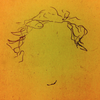
bristel
1,252 PointsAwesome, thank you! I should have known that! We haven't learned ternary operators yet but I'll research it! :)