Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial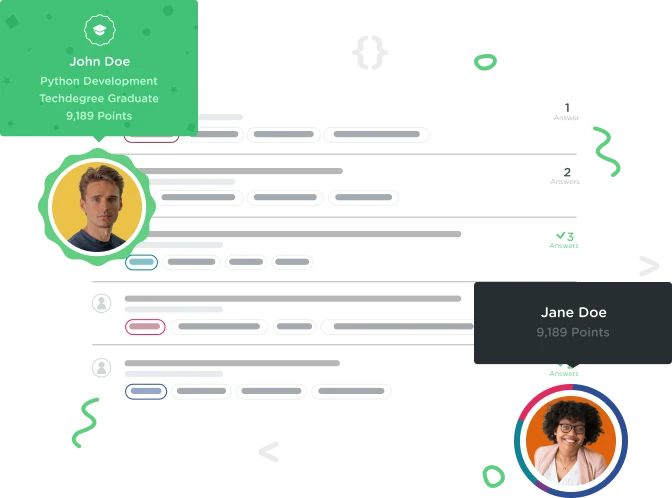

mfod
1,708 PointsNot sure why dispense() method has wasDispense = true.
public boolean dispense() {
boolean wasDispensed = false;
if (!isEmpty()) {
mPezCount--;
wasDispensed = true; //why is this set to true if it will return false anyway?
}
return wasDispensed;
}
Is it returning true or false? If its returning false then why set it to true inside the if statement? What purpose does that have?
4 Answers
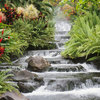
kabir k
Courses Plus Student 18,036 PointsHi Mariam,
Like Harry pointed out, there are two outcomes that you could expect from the dispense() method -
1) when the dispenser is empty and
2) when the dispenser isn't empty
and bear in mind that the dispenser method has a boolean return type i.e. it returns true or false either way.
So in the first line of the dispense() method's code block,
boolean wasDispensed = false;
the code shows that at the start, the local variable, wasDispensed will hold the boolean value of false (because no Pez has been dispensed yet)
Then we say, using the if statement, if it is not empty, remove a Pez by decrementing it by 1 with this piece of code
mPezCount--;
Then the local variable, wasDispensed is now assigned, true (because a Pez has now been dispensed) as shown by this code
wasDispensed = true;
Then finally, the dispense method will return true to show us that it has performed its function of dispensing a Pez. This is for case 2) for when the dispenser is not empty.
For case 1) on the other hand, if the dispenser is empty, the if statement is not gonna run and the dispense() method will simply return the default value of the local variable, wasDispense, which is false telling us that no Pez was dispensed by the method.
I hope this helps.
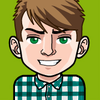
Harry James
14,780 PointsHey Mariam,
It might help to read this out step by step to see what's going on:
- Set wasDispensed to false.
- If the Pez Dispenser isn't empty, go ahead and dispense a Pez, then set wasDispensed to true.
- Finally, return the value of wasDispensed.
You can then go through these 3 steps in your head - think about the value of wasDispensed under two scenarios - when the pez dispenser is empty and when the pez dispenser isn't empty.
Hopefully this should explain what's going on in the code for you but if there's still something you don't quite understand, give me a shout :)

Andrew Winkler
37,739 Pointspublic boolean dispense() { // declare a new method with the return type boolean
boolean wasDispensed = false; // set the boolean "wasDispensed" to "false"
// ^^^ THIS CODE BLOCK will be skipped if the dispenser is empty
if (!isEmpty()) { // if the dispenser IS NOT empty, this code block will run.
mPezCount--; // subtract 1 from the mPezCount variable (symbolizing 1 pez being dispensed)
wasDispensed = true; // set the "wasDispensed" variable to the value of "true" (symbolizing 1 pez being dispensed)
}
return wasDispensed; // returns a boolean indicator if 1 pez was dispensed
}
Why did he program it like this? Idk, I would've made isEmpty the boolean and made block mPezCount == 0 { isEmpty = True;} statement that would have ran after the count was decremented, but considering object oriented monogramming and the rules of best practice, his logic works roughly as well.
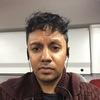
Akash Sharma
Full Stack JavaScript Techdegree Student 14,147 Pointsso are we needing the wasDispensed variable anywhere like after we call dispenser.dispense method and we subtract 12 pez candies then mPezCount should equal 0.
So now lets call the dispenser.dispense method again but now it will return the wasDispensed to false value would anything come up on the console? Or are we keeping this variable to use it somewhere else?
Also what if we wanted to rewrite the method so we pass in how many pez to dispense 0 - 12 as in argument inclusive would we still call it a boolean method ?