Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial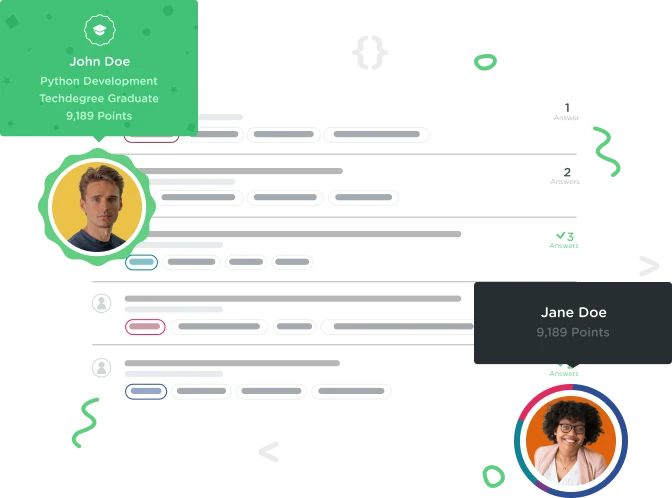

Peng Ren
4,119 Pointsnot sure why I have an EOF problem
def disemvowel(word): word = input() list(word) for letter in word: while letter.lower() == 'a' or letter.lower() == 'e' or letter.lower() == 'i' or letter.lower() == 'o' or letter.lower() == 'u': word.remove(letter) join(word) return word
this is my code, it kept saying EOFError, not sure why
def disemvowel(word):
word = input()
list(word)
for letter in word:
while letter.lower() == 'a' or letter.lower() == 'e' or letter.lower() == 'i' or letter.lower() == 'o' or letter.lower() == 'u':
word.remove(letter)
join(word)
return word
2 Answers
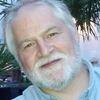
Jeff Muday
Treehouse Moderator 28,723 PointsNice try-- conceptually, I think you have a good understanding of the challenge... let's build on that.
I am suggesting some changes and explain why the change needs to be made.
- 1 comment out "word = input()"
Since you passed in the parameter word-- we don't want to override it. Comment that out.
- 2 CHANGE "list(word)" to "word = list(word)"
"list(word)" creates a new list from the string "word", but it has to be assigned on the left hand side to the word variable since strings are immutable.
- 3 ITERATOR should be a copy of original word
If you remove elements from the word list during a "for" loop, it can have unexpected results -- it is recommended to work on a copy of the list. That's easy enough to fix using "word.copy()"
- 4 REMOVE "while" REPLACE with "if"
I can tell you intended to use an "if" statement to check the presence of a vowel. Using a while loop sounds great but would cause an infinite loop condition causing your EOF error.
- CHANGE "join"
the join() is a string method so it requires a string to join inbetween each list element. In this case it is a simple quote-quote or empty string.
def disemvowel(word):
#word = input() # 1
word = list(word) # 2
for letter in word.copy(): # 3
# 4 removed "while" on conditional below.
if letter.lower() == 'a' or letter.lower() == 'e' or letter.lower() == 'i' or letter.lower() == 'o' or letter.lower() == 'u':
word.remove(letter)
''.join(word) # 5
return word
# now we can test it out.
print(disemvowel('I am disemvoweled'))
The new code will yield the correct output.
['d', 's', 'm', 'v', 'w', 'l', 'd']
I hope this helps!

Peng Ren
4,119 Pointsgot it, thank you!