Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial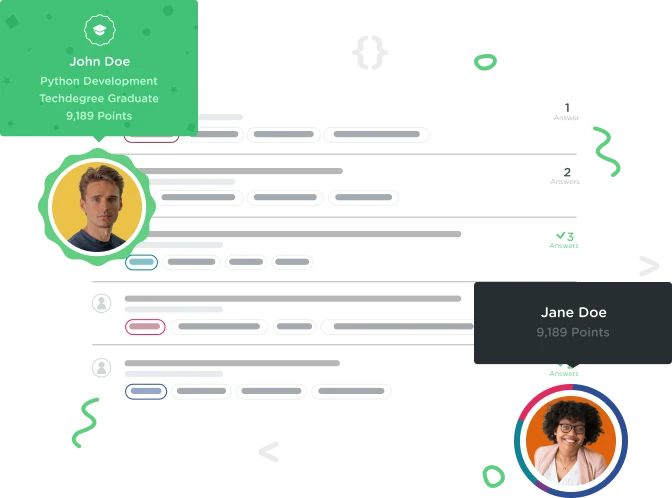

diegoholt
1,076 PointsNot sure why I'm not getting the right answer.
Can someone take a look at my code and let me know what I'm doing incorrectly?
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
parsed_int = int(num)
except ValueError:
return num
return parsed_int * parsed_int

diegoholt
1,076 Pointsahh, misread the direction. Thanks guys!
1 Answer

Cindy Lea
Courses Plus Student 6,497 PointsHere is another way to approach it:
EXAMPLES
squared(5) would return 25
squared("2") would return 4
squared("tim") would return "timtimtim"
def squared(a): try: a = int(a) a = a * a except ValueError: a = a * len(a) return a
Russell Sawyer
Front End Web Development Techdegree Student 15,705 PointsRussell Sawyer
Front End Web Development Techdegree Student 15,705 PointsThe challenge is asking you to multiply a number by itself, or square the number, and return the value. If the number introduced to the function can't be converted to an integer, meaning it's a string, then return the string the same amount of times as the letters in the string.
Keep in mind you need to return a value in the try: section
You also only need one return value in the except ValueError: section