Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial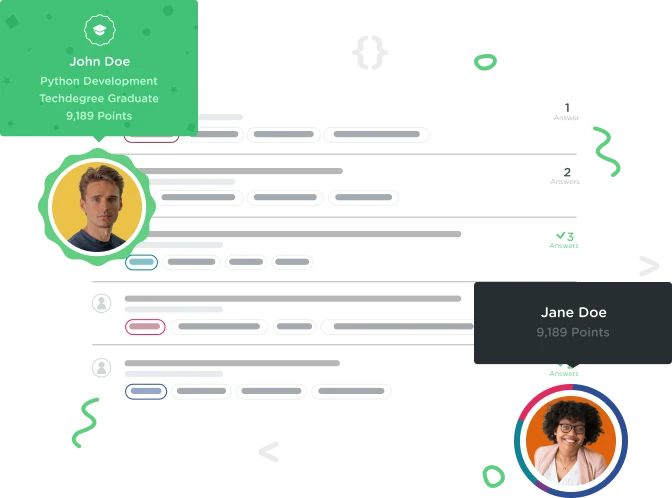

Anthony Lopez
963 PointsNot sure why my code isn't working!
I'm currently working on the problem of removing vowels from a word. What I did was turn the string into a list and removed the vowels using .remove(). I typed it all out in my desktop python shell and it worked just fine, but when I copied it into the teamtreehouse workspace to be checked it kept saying that it expected an answer but received the word none. Is there something wrong with it?
def disemvowel(word): word_list = list(word) try: word_list.remove("a") except ValueError: pass try: word_list.remove("e") except ValueError: pass try: word_list.remove("i") except ValueError: pass try: word_list.remove("o") except ValueError: pass try: word_list.remove("u") except ValueError: pass try: word_list.remove("A") except ValueError: pass try: word_list.remove("E") except ValueError: pass try: word_list.remove("I") except ValueError: pass try: word_list.remove("O") except ValueError: pass try: word_list.remove("U") except ValueError: pass word = "".join(word_list) print(word)
p.s. I couldn't figure out how to incorporate .upper() or .lower() or how to incorporate return. Would love some advice on how to make this solution smaller and cleaner! Thanks.
def disemvowel(word):
word_list = list(word)
try:
word_list.remove("a")
except ValueError:
pass
try:
word_list.remove("e")
except ValueError:
pass
try:
word_list.remove("i")
except ValueError:
pass
try:
word_list.remove("o")
except ValueError:
pass
try:
word_list.remove("u")
except ValueError:
pass
try:
word_list.remove("A")
except ValueError:
pass
try:
word_list.remove("E")
except ValueError:
pass
try:
word_list.remove("I")
except ValueError:
pass
try:
word_list.remove("O")
except ValueError:
pass
try:
word_list.remove("U")
except ValueError:
pass
word = "".join(word_list)
print(word)
2 Answers
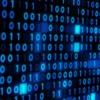
Alexander Davison
65,469 PointsThe remove
function removes only the first
find of the given argument.
For example: (Pretend I'm in the Python Shell)
>>> my_list = [1, 2, 3]
>>> my_list.remove(2)
>>> my_list
[1, 3]
>>> my_new_list = [1, 2, 3, 2, 1]
>>> my_new_list.remove(2)
>>> my_new_list
[1, 3, 2, 1]
I hope this helps!
~Alex

Ken Alger
Treehouse TeacherAnthony;
As you noted, your function is returning anything, which is causing it to not pass the challenge. The challenge checking engine will call, for example disemvowel("hello")
and is expecting to get "hll" returned.
In terms of writing your code is a simpler, cleaner, fashion, I would recommend using a for
loop with an if
statement. Inside that if statement you can manage the results of the letter checking process. Then return the results.
Have another look at it with that in mind and post back if you're still stuck.
Happy coding,
Ken

Anthony Lopez
963 PointsThanks for the suggestion Ken I will work on incorporating the for loop!
That's actually what I'm confused about because I just called hello in the IDLE for python and it returned hll. If I could post images I could show you that it works!
But for some reason when I do the same thing in the workspace here it's not able to execute properly. I'm just curious why that is.

Ken Alger
Treehouse TeacherNot sure why it wouldn't work in Workspaces. If you're talking about the challenge itself, the checking engine gets pretty picky sometimes. And, as I mentioned, your functions isn't/wasn't returning anything for it to examine. ;-)

Anthony Lopez
963 PointsKen! I finally got the hint (return).
Thank you very much haha.
Anthony Lopez
963 PointsAnthony Lopez
963 PointsHey thanks for your reply Alex!
The issue is actually that my code that works in my python shell is not working in the teamtreehouse workspace.
The conditions for the challenge are totally met within the shell.
For example when I call disemvowel(NoQIaAmuE)
I will get NQm.
But when I run it in the teamtreehouse workspace I get none.